I’ve whipped up a quick module which allows you to tween models with just a single function and some content
data.
The module scripts consists of:
-- services
local tweenService: TweenService = game:GetService("TweenService") :: TweenService
-- variables
local defaultTweenInfo: any = {
Time = 1,
EasingStyle = "Sine",
EasingDirection = "Out",
RepeatCount = 0,
Reverses = false,
DelayTime = 0,
}
-- functions
return function(model: Model, content: any)
local cframeValue: CFrameValue = Instance.new("CFrameValue") :: CFrameValue
cframeValue.Value = model:GetPivot()
local cframeChangedConection: RBXScriptConnection = cframeValue:GetPropertyChangedSignal("Value"):Connect(function()
model:PivotTo(cframeValue.Value)
end) :: RBXScriptConnection
local cframeChangeTween: Tween = tweenService:Create(
cframeValue,
TweenInfo.new(
content.TweenInfo.Length :: number,
Enum.EasingStyle[content.TweenInfo.Style] :: Enum.EasingStyle,
Enum.EasingDirection[content.TweenInfo.Direction] :: Enum.EasingDirection,
content.TweenInfo.Repeats :: number,
content.TweenInfo.Reverse :: boolean,
content.TweenInfo.Delay :: number
),
content.Properties
)
cframeChangeTween:Play()
cframeChangeTween.Completed:Wait()
cframeChangedConection:Disconnect()
cframeValue:Destroy()
end
The module simply creates a new CFrameValue
and then tweens the Value
property of it. Then a signal detects when a change is made to the CFrameValue
and then uses the :PivotTo
method to move the actual model ( As others have mentioned above I believe )
This module is great because it means you don’t have to go out of your way to weld anything. The module also accommodates for float point problems. This means you won’t have tiny gaps beween the parts in the model over extensively using the function; Perfect for a train system:
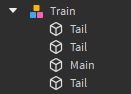
The video is an example of a train which just moves on a straight line. Of course this train could be anything, a car, boat, roller coaster or even a penguin! And it can certainly move in more ways than just a straight line!
Now, the LocalScript
consists of a very simple call of the function:
-- services
local replicatedStorage: ReplicatedStorage = game:GetService("ReplicatedStorage")
-- modules
local tweenModel: any = require(replicatedStorage:WaitForChild("Tween Model", 100) :: ModuleScript) :: any
-- functions
tweenModel(workspace.Train, {
TweenInfo = {
Length = 5,
Style = "Sine",
Direction = "Out",
Repeats = 0,
Reverse = false,
Delay = 6,
},
Properties = {
Value = CFrame.new(46, 6.75, 1.5),
},
})
The second argument you see in the tweenModel
function is the content
data I mentioned earlier. It consists of two other tables within it. Which of being TweenInfo
and Properties
. The TweenInfo
table controls how the model will actually move.
Tweeninfo table
- The
Length
is how long the tween takes.
- The
Style
is how it moves from point a
to point b
( here is a link to view the different styles )
- The
Direction
is how the style is converge from point a
to point b
( here is a link to view the different directions )
- The
Repeats
is how many times the tween will repeat.
- The
Reverse
is if the tween is supposed to reverse after it has been completed.
- The
Delay
is how much time passes before playing the tween.
Properties table.
The properties table only contains one value ( which is to be fed in ) This value is called, well… Value
. This is the value that the CFrameValue
will use to tween using the TweenInfo
data.
I hope this helped even a little in your endeavour of creating your roller coaster project.