I am doing this commission where I am making a therapy center and when you join it’s not supposed to spawn your character and you get to choose a team with a camera tween in the background but it’s not working. Instead what happens is that the UI turns invisible and the camera doesn’t move and it looks like this .
Here is a screenshot of my workspace.
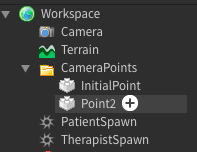
Here is a screenshot of the “Teams” service.
Here is a screenshot of my (server-side) script and remote function
And finally here is a screenshot of my UI.
Here is the code for the “Main” local script (third screenshot).
local menu = script.Parent.Menu
local welcome = script.Parent.Welcome
local therapist = menu.Therapist
local patient = menu.Patient
local camera = workspace.CurrentCamera
local initialPoint = workspace.CameraPoints:WaitForChild("InitialPoint")
local point2 = workspace.CameraPoints:WaitForChild("Point2")
local teamChosen = game.ReplicatedStorage.teamChosen
function tween()
local tweenService = game:GetService("TweenService")
camera.CameraType = Enum.CameraType.Scriptable
camera.CFrame = initialPoint.CFrame
camera.Focus = point2.CFrame
local tweenInfo = TweenInfo.new(
20,
Enum.EasingStyle.Linear,
Enum.EasingDirection.Out,
0,
false,
0
)
local myTween = tweenService:Create(camera,tweenInfo,{CFrame = point2.CFrame})
myTween:Play()
print("Tweened Played")
end
tween()
wait(10)
therapist.MouseButton1Click:Connect(function()
teamChosen:FireServer("Therapist")
camera.CameraType = "Custom"
menu.Visible = false
welcome.Visible = false
end)
patient.MouseButton1Click:Connect(function()
teamChosen:FireServer("Patient")
camera.CameraType = "Custom"
menu.Visible = false
welcome.Visible = false
end)
Finally here is the code for the “onTeamChosen” script.
local teamChosen = game.ReplicatedStorage.teamChosen
teamChosen.OnServerInvoke = function(player,team)
print("Invoked")
local selectedTeam = game:GetService("Teams"):FindFirstChild(team)
player.Team = selectedTeam
player:LoadCharacter()
return
end
A thought about what the problem could be with…
I think it might have something to do with returning in the (server side) onTeamChosen script.