The whole script works fine other than the part that is supposed to make the UI visible - no errors shown.
local ClickDetector = script.Parent.ClickDetector
local Label = script.Parent.SurfaceGui.Frame.TextLabel
ClickDetector.MouseClick:Connect(function(Player)
local username = Player.Name
if Label.Text == "Unclaimed" then
if not game.Workspace:FindFirstChild("Claimed: "..username) then
Label.Text = username
script.Parent.Parent.Name = "Claimed: "..username
elseif Label.Text == username then
Player:WaitForChild("PlayerGui").ScreenGui.Frame.Visible = true
else
print("You already have a mat!")
end
end
end)
Can you show me a screenshot of your hierarchy? “ScreenGui” Isnt a specifically named thing so you might be referencing the wrong one, same with “Frame”
This is the only one I’ve put in so far.
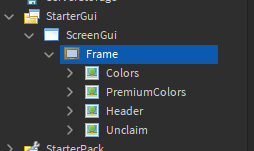
Where exactly is your script located?
It’s inside of a part in the workspace.
I am not 100% sure if this will fix the issue, but worth a shot:
Consider removing “WaitForChild” and simply put: Player.PlayerGui.ScreenGui.Frame.Visible = true. WaitForChild is not typically needed for scripts handled on the server (in most cases).
Try writing it as this:
Player.PlayerGui:WaitForChild("ScreenGui").Enabled = true -- ignore this if its already true
Player.PlayerGui:WaitForChild("ScreenGui"):WaitForChild("Frame").Visible = true -- also check if you set transparency not visiblity
Hm, this doesn’t seem to be working either, I may just make a separate script for it and see if that fixes anything
Question: Is this a Server or Local Script?
use a remote event as its much less complicated…
since its a server script you gotta use remote event
Try this perhaps and see what this outputs? (Also keep in mind if you’re changing the text from the client side, the server won’t be able to detect it)
local ClickDetector = script.Parent.ClickDetector
local Label = script.Parent.SurfaceGui.Frame.TextLabel
ClickDetector.MouseClick:Connect(function(Player)
local username = Player.Name
local Frame = Player.PlayerGui:WaitForChild("ScreenGui").Frame
print(Frame.Visible)
print(Label.Text)
if Label.Text == "Unclaimed" then
if not game.Workspace:FindFirstChild("Claimed: "..username) then
Label.Text = username
script.Parent.Parent.Name = "Claimed: "..username
elseif Label.Text == username then
Player:WaitForChild("PlayerGui").ScreenGui.Frame.Visible = true
else
print("You already have a mat!")
end
end
end)
@Creeperman16487 A ClickDetector is fine with referencing the Player as a parameter, the only difference is just a slight lag delay so there’s no need for using a RemoteEvent
Yea this is having the same issue as the original script, although I tried removing it from the main script all together and made a secondary script, and it is working fine now
I removed the section that wasn’t working from the main script and made it it’s own script in the same part, now it’s working fine. Although I’m still not sure why the original script wouldn’t work, if anyone knows why I’d really appreciate it if you explained!
Main Script:
local ClickDetector = script.Parent.ClickDetector
local Label = script.Parent.SurfaceGui.Frame.TextLabel
ClickDetector.MouseClick:Connect(function(Player)
local username = Player.Name
if Label.Text == "Unclaimed" then
if not game.Workspace:FindFirstChild("Claimed: "..username) then
Label.Text = username
script.Parent.Parent.Name = "Claimed: "..username
elseif Label.Text == username then
print("Mat settings should be open.")
else
print("You already have a mat!")
end
end
end)
Second Script:
script.Parent.ClickDetector.MouseClick:Connect(function(player)
local username = player.Name
if script.Parent.SurfaceGui.Frame.TextLabel.Text == username then
player:WaitForChild("PlayerGui").ScreenGui.Frame.Visible = true
end
end)