My gun tool doesn’t activate when the corresponding key is pressed
The output doesn’t show anything either, I don’t get any errors when pressing keys
I couldn’t find any solutions for this
userInputService.InputBegan:Connect(function(input)
if input.KeyCode == Enum.UserInputType.MouseButton1 and isEquipped == true then
local Anim = Instance.new("Animation")
Anim.AnimationId = "rbxassetid://16700037866"
local track
track = script.Parent.Parent.Humanoid:LoadAnimation(Anim)
track.Priority = Enum.AnimationPriority.Action4
track.Looped = false
track:Play()
end
end)
It should just play the animation when MouseButton1 is pressed, but it does nothing, the animation also has priority above every other animation
Where my script is:
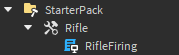
local isEquipped = true -- Assuming you set this elsewhere
userInputService.InputBegan:Connect(function(input)
if input.KeyCode == Enum.UserInputType.MouseButton1 and isEquipped then
print("MouseButton1 pressed")
local Anim = Instance.new("Animation")
Anim.AnimationId = "rbxassetid://16700037866"
local track = script.Parent.Parent.Humanoid:LoadAnimation(Anim)
track.Priority = Enum.AnimationPriority.Action4
track.Looped = false
track:Play()
print("Animation started")
end
end)
use Tool.Activated it is more reliable and shortens the code. And it works for both pc and mobile
This doesnt seem to do anything still, no output either
I want to add reloading/crouching etc. to the tool, i can use Tool.Activated for the firing but i need UIS for the other functions
-
Input Service Connection:
-
Check the isEquipped
Variable:
- Confirm that the
isEquipped
variable is correctly set to true
when the gun tool is equipped. If it’s not set properly, the animation won’t play even if the MouseButton1 is pressed.
-
Animation Loading and Playing:
-
Animation Priority and Conflicts:
- Ensure that there are no conflicting animations with higher priorities that might interrupt or override this one.
- Check if any other animations are playing simultaneously and interfering with this one.
-
Debugging Output:
Let me know if you have any furher issues.
I have done all of this, the animations work fine as ive tested them outside of using UIS with the same code
full code
local userInputService = game:GetService("UserInputService")
local tool = script.Parent
local plr = game.Players.LocalPlayer
local character = plr.CharacterAdded:Wait()
local humanoid = character:WaitForChild("Humanoid")
local isEquipped = false
local reloading = false
local firing = false
local crouching = false
tool.Equipped:Connect(function()
isEquipped = true
end)
tool.Unequipped:Connect(function()
isEquipped = false
end)
userInputService.InputBegan:Connect(function(input)
if input.KeyCode == Enum.UserInputType.MouseButton1 and isEquipped then
print("MouseButton1 pressed")
local Anim = Instance.new("Animation")
Anim.AnimationId = "rbxassetid://16700037866"
local track = script.Parent.Parent.Humanoid:LoadAnimation(Anim)
track.Priority = Enum.AnimationPriority.Action4
track.Looped = false
track:Play()
print("Animation started")
end
end)
This still does not work, and wont print anything either
I did some change to help debug. But I cant get sure if the animation is playing right.
local userInputService = game:GetService("UserInputService")
local isEquipped = true -- Make sure this is set correctly
userInputService.InputBegan:Connect(function(input)
if input.UserInputType == Enum.UserInputType.MouseButton1 and isEquipped then
print("MouseButton1 pressed")
local Anim = Instance.new("Animation")
Anim.AnimationId = "rbxassetid://16700037866"
local track
local success, error = pcall(function()
track = script.Parent.Parent.Humanoid:LoadAnimation(Anim)
end)
if success then
track.Priority = Enum.AnimationPriority.Action4
track.Looped = false
track:Play()
print("Animation played successfully")
else
print("Error loading animation:", error)
end
end
end)
I tried this, it still doesnt print anything, the isEquipped variable is definitely set as True and im pressing M1
I tried placing it in starterCharacterScripts but that still doesnt output, maybe it has to do with the place im running it in?
Are you sure you edit the right file? Can you make the rifle print hello?
If you change your code for:
local userInputService = game:GetService("UserInputService")
userInputService.InputBegan:Connect(function(input)
print('Hello')
end)
You get the “Hello” right?
It wont print hello, what file does it need to be? i have it as a localscript as a child of the tool
Yes. Folder is StarterCharacterScripts , create a Tool , create a localscript inside the tool. Try to create another tool.
Okay, creating a new tool lets it print “hello” not sure why it doesnt work with the rifle though, im using the exact same code (the rifle prints hello, but only when there is no other code aside from the printing)
Try to delete the rifle and rename the new tool.
So, this:
local userInputService = game:GetService("UserInputService")
local plr = game.Players.LocalPlayer
local character = plr.CharacterAdded:Wait()
local humanoid = character:WaitForChild("Humanoid")
local isEquipped = true
local crouching = false
userInputService.InputBegan:Connect(function(input)
if input.KeyCode == Enum.KeyCode.LeftControl and isEquipped == true then
local Anim = Instance.new("Animation")
Anim.AnimationId = "rbxassetid://16706440839"
local track
track = script.Parent.Parent.Humanoid:LoadAnimation(Anim)
track.Priority = Enum.AnimationPriority.Action4
track.Looped = false
if crouching == true then
crouching = false
humanoid.WalkSpeed = 16
track:Stop()
else
humanoid.WalkSpeed = 4
crouching = true
track:Play()
task.wait(track.Length * 0.98)
track:AdjustSpeed(0)
end
end
end)
Doesnt work (does nothing), but this:
local userInputService = game:GetService("UserInputService")
userInputService.InputBegan:Connect(function(input)
print('Hello')
end)
Outputs “hello”
Both codes are missing the
local userInputService = game:GetService(“UserInputService”)
Oh sorry, i formatted the code block wrong, they both have that statement