Hi, I want to check if a bool is true/false in an attribute and check numbervalues as an attribute. I’ve tried to achieve that:
local FourGame = workspace.FourCC
local DirectHeatLevel = FourGame.ReactorStats:GetAttribute("DirectHeatButtonLevel") --numbervalue
local DirectHeatBool = FourGame.ReactorStats:GetAttribute("Heat") --boolvalue
if DirectHeatLevel => 5 then
print("five")
end
if DirectHeatBool == true then
print("hi")
end
None of the code results in any result. I’m not sure if I should resort back to values or stick with attributes. Any help would be appreciated.
I believe that =>
is causing an error when you’re checking for the value of DirectHeatLevel
– in order to resolve this, the operator needs to be flipped the other way around so that it’s >=
instead of =>
.
If nothing ends up printing after that, if you’re modifying the attributes from a script, you could add alternate conditions to see if there isn’t an attribute called DirectHeatButtonLevel
or Heat
along with the case where those values don’t match the conditions you’ve setup.
Oh, okay… Yeah, I’m gonna fix that.
However, the bool for the attribute doesn’t print anything. Is there an issue I’m doing for that?
The second condition won’t be checked for until the error above has been resolved. Unless you’ve already fixed the =>
in the first conditional statement and tested to see if it works with no errors, you could try printing the value of DirectHeatBool
to see if it’s false, nil, etc.
The check seems like it always results in “false” when it’s being put in a while true do loop, even though the bool/numbervalue meets it’s expectations.
local FourGame = workspace.FourCC
local DirectHeatLevel = FourGame.ReactorStats:GetAttribute("DirectHeatButtonLevel") --numbervalue
local DirectHeatBool = FourGame.ReactorStats:GetAttribute("Heat") --boolvalue
while true do
wait(1)
if DirectHeatBool == true and DirectHeatLevel >= 5 then
print("yeah")
else
print("nah")
end
end
Try printing the actual values of the attributes since you’ve already determined that the condition you’re looking for isn’t being met. This will allow you to see if the Attribute has a value set in the first place (it’ll return a non-nil value), or if there’s simply a different value than what you’re looking for.
print(DirectHeatBool)
print(DirectHeatLevel)
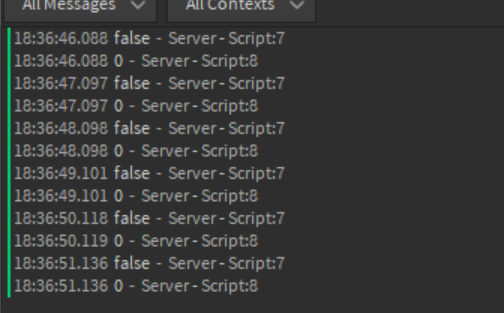

Yeah… I’m not sure if they print the current values.
If you’re changing the values of those attributes during runtime, whether manually or from any kind of script, make sure it’s not being done from the client if you’re checking for the new values from the server.
From my understanding, the server won’t be able to see the changes that the client made to each attribute without additional communication.
I’m doing the test on the server’s side in runtime, but it doesn’t work no matter what 
In that case, because the variable is only being updated upon the script running for the first time, whenever it’s accessed later on in the script, the updated value of the attribute isn’t showing.
Try this to see if it ends up producing any different results:
local FourGame = workspace.FourCC
local ReactorStats = FourGame.ReactorStats
local count = 1
local function checkAttributeValues() -- Whenever the function is activated, the two variables will be updated with the current values of the attributes
print("This function has been activated "..count.." time(s)")
count += 1
local DirectHeatLevel = ReactorStats:GetAttribute("DirectHeatButtonLevel")
local DirectHeatBool = ReactorStats:GetAttribute("Heat")
print(DirectHeatLevel)
warn(DirectHeatBool)
end
while true do
wait(2)
checkAttributeValues()
end