That is some interesting behavior. I think you got the directions of one of your vectors wrong. vec1
should be a left-facing vector relative to the rootpart, assuming of course that your character is standing up straight, but in that case the Region3 should still work. Have you verified that the base vectors are what you’re expecting them to be?
However, there are easier and less error-prone ways to achieve what you’re trying to do. I suggest you read the wiki page for CFrames as well as the tutorial on understanding CFrames for more information.
You can actually get the upwards, right-facing and backwards unit vectors from the CFrame itself. A CFrame is essentially nothing more than a rotation matrix with a position, and a rotation matrix in 3D space is essentially nothing more than three vector3s giving those directions. You can get the numbers making up all four vectors (directions and the position) using CFrame:components()
:
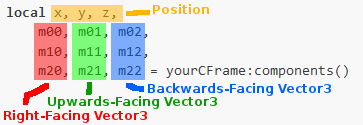
To put this into code:
local x, y, z,
m00, m01, m02,
m10, m11, m12,
m20, m21, m22 = yourCFrame:components()
local position = Vector3.new(x, y, z)
local right = Vector3.new(m00, m10, m20)
local up = Vector3.new(m01, m11, m21)
local back = Vector3.new(m02, m12, m22)
From there you can get any of the corners you want. For example:
local topRight = position + 4*right - 7*back + 10*up
local bottomLeft = position - 2*right + 2*back
However, it can get even simpler than that. CFrames actually have pre-made methods to do these spatial transformations for you. In your case, you’d want to look into CFrame:pointToWorldSpace()
, which takes a Vector3 position relative to that CFrame and converts it into a Vector3 position in the world. For example:
local topRight = yourCFrame:pointToWorldSpace(Vector3.new(4, 10, -7))
local bottomLeft = yourCFrame:pointToWorldSpace(Vector3.new(-2, 0, 2))
Note that the X
direction is to the right, the Y
direction is upwards and the Z
direction is backwards (not forwards).
Hopefully one of these approaches will help you solve your problem. 
EDIT:
This might actually be a better approach to the problem, because using coordinates relative to the player causes the Region3 to have a different size depending on the orientation of the player.