I’m trying to achieve sway affecting the weapon when it it holstered onto the player’s character. So it sways every time the character moves.
I’ve achieved this using tween service and the c0 property of a motor6D and it works fine. But I want to transition to spring module to make it more natural and fluid but have no idea how to use it.
This is the script so far:
function CancelAllTweens()
for i,v in pairs(Tweens) do
table.remove(Tweens, i)
v:Cancel()
v:Destroy()
end
return
end
local Model = script.Parent
local Char = Model.Parent
local Weld02 = Model:WaitForChild("Motor6D")
game:GetService("RunService").Stepped:Connect(function()
if Char.Torso.Velocity.X < 0 or Char.Torso.Velocity.Y < 0 or Char.Torso.Velocity.Z < 0 then
local Tween01 = game:GetService("TweenService"):Create(Weld02, TweenInfo.new(0.05), {C0 = CFrame.Angles(math.rad(0), math.rad(0), math.rad(10))})
CancelAllTweens()
table.insert(Tweens, Tween01)
Tween01:Play()
elseif Char.Torso.Velocity.X > 0 or Char.Torso.Velocity.Y > 0 or Char.Torso.Velocity.Z > 0 then
local Tween02 = game:GetService("TweenService"):Create(Weld02, TweenInfo.new(0.05), {C0 = CFrame.Angles(math.rad(0), math.rad(0), math.rad(-10))})
CancelAllTweens()
table.insert(Tweens, Tween02)
Tween02:Play()
else
local Tween = game:GetService("TweenService"):Create(Weld02, TweenInfo.new(0.01, Enum.EasingStyle.Sine), {C0 = CFrame.Angles(math.rad(0), math.rad(0), math.rad(0))})
CancelAllTweens()
table.insert(Tweens, Tween)
Tween:Play()
end
end)
If you could give me tips or tell me how to use the physics based spring module to achieve this i would greatly appreciate it.
2 Likes
if you want to make it physics based, why use a script at all? You could attach two parts to the torso of the player, then a part attached to two springs and prismatic constraints between the two other parts, then attach the holster again with a hinge (I’ll draw something to make that make sense, since I explain things bad, lol.)
1 Like
If you are talking about bhristt’s module, I’m afraid I don’t have experience with that. However, I can guide you on how to make sway with Quenty’s spring module
Let me rephrase, you’d use a script, but for the bouncing of the holster during a jump or something, I don’t see a need for a module for that. (Though I’m inexperienced with physics based stuff in animations, so idk)
And here’s an explanation of what I meant before:
Green = The two parts the blue is springing off of.
Blue = The part that adds the springing effect by having springs attached to it. The prismatic constraints just keep it in place on the other two axes.
Orange = Prismatic constraints
Purple = Spring constraints
Reddish-Purplish = Hinge, so the holster can swing freely on the relative X axis. You could switch this out for a ball socket constraint if you want it to swing freely on the relative X and Z axis both.
I’m not sure if this’d actually work, because I’m not sure that the motion of the torso would actually be converted into spring behavior, but I think it’d be cool and look good if it did work.
1 Like
Yea i don’t mind whichever spring module works i’ll try it out, it doesnt have to be a specific one
I tried using spring constraints but for some reason the character started glitching out whenever i used it in the game even though i set the weapon to massless. It was if the springs were weighing the character down but not slowing it down.
Like this:
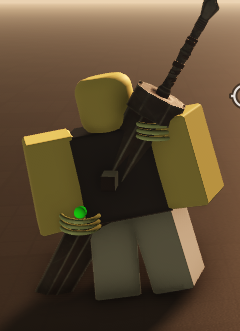
See how its tilting a little bit to the left. It does this jittery movement.
I also tried rope constraints and it had the same effect
1 Like
The issue with springs like this in roblox is they can be very annoying sometimes in terms of messing with other thing’s physics, try making sure the spring stiffness is high, and try different configurations of properties. Also make sure the prismatic constraint’s limits are larger than the max length of the spring, or else they’ll glitch things too. It might not work with a character, cause it might cause too much glitching.
The reason I suggested it was just cause I’ve been working on fully physics based things lately and thought it could work.
Also set collisions properly too, the green and blue parts can have cancollide off, aswell as being transparent.
2 Likes
wait nvm i kind of did it using your method but more simplified. I think you were talking about a different type of holster to what i meant lol.
I just meant having the weapon attached to your back and have it swing about instead of stationary. Anyways i attached to springs to attachments on the sword and two on parts connected to the torso and now it works.
I found out the jitter was caused by some tilt effect local script i made which idk why it messes up with the spring constraints but yeah it sort of works now. There is an occasional jitter though but at least it doesn’t happen 100% of the time
1 Like
Yeah i had to set the max length higher because the spring would bring the weapon too close to the player causing it to mess with it’s movements and now it’s fixed ty
1 Like
Oh! good you got it working then. I was talking about a gun holster, yeah, but whatever works works!
Could you show a video of how it looks? I’m actually interested in how smooth it will be
I made a few tweaks and switched to BallSocketConstraints because i can limit the angle in which the weapon moves. But i’m still trying to limit it, since as you can see in the video the weapon spins way too much and too quickly so limiting it is important for the realism.
1 Like
Ooh, I like it already. Yeah, with limiting it’d look amazing!
1 Like
I believe the effect can be better achieved with springs tbh. But I’m unsure of what you mean by sway. I had assumed it would mean the weapon would move slightly in the direction the character is moving but seems like the weapon should turn???
1 Like
Well my aim is to get a slight movement. But i don’t want it to twist like how it is in the video. You see how it is spinning rapidly especially towards the end. I don’t want that part. I only want slight movement like in the start.
1 Like
I think @Mystxry12 and me both were under the assumption that you meant sway as in moving the the left and right of the character in space, not sway as in the weapon turning slightly while in the same position, but honestly the effect you’re going for is cool too.
Personally I’d add both effects, could create a very fluid system. But looks good now regardless.
1 Like
You would achieve the same result but smoother imo with Springs with something like this:
local Spring = require(...)
local Camera = workspace.CurrentCamera
local weaponSway = Spring.new()
weaponSway.Damper = 0.8 --// Modify (lower value = more springy)
weaponSway.Speed = 8 --// Modify (higher value = spring will reach target in less time
local lastCameraRotation = 0
RunService.RenderStepped:Connect(function(delta)
local _, yRotation, _ = Camera.CFrame:ToOrientation()
weaponSway:Impulse((yRotation - lastCameraRotation) * delta * 10)
weapon.CFrame *= CFrame.Angles(0, -weaponSway.Position, 0)
end)
Yea not sure this is meant to be happening lol
This is the script:
local Weapon = script.Parent -- WeaponModel
local WeaponModel = Weapon.Model --WeaponMeshPart
local Char = Weapon.Parent
local Weld02 = Weapon:WaitForChild("Motor6D")
print(WeaponModel.Position)
local Camera = workspace.CurrentCamera
local weaponSway = Spring.new()
weaponSway.Damper = 0.8 --// Modify (lower value = more springy)
weaponSway.Speed = 8 --// Modify (higher value = spring will reach target in less time
local lastCameraRotation = 0
RunService.Heartbeat:Connect(function(delta)
local _, yRotation, _ = Camera.CFrame:ToOrientation()
weaponSway:Impulse((yRotation - lastCameraRotation) * delta * 10)
WeaponModel.CFrame *= CFrame.Angles(0, weaponSway.Position, 0)
end)
This is using [Quenty’s spring module] right?
Oh god I forgot that motor6ds were included
(I suck at those). Try this and lmk if it works.
local Weapon = script.Parent -- WeaponModel
local WeaponModel = Weapon.Model --WeaponMeshPart
local Char = Weapon.Parent
local Weld = Weapon:WaitForChild("Motor6D")
print(WeaponModel.Position)
local Camera = workspace.CurrentCamera
local weaponSway = Spring.new()
weaponSway.Damper = 0.8 --// Modify (lower value = more springy)
weaponSway.Speed = 8 --// Modify (higher value = spring will reach target in less time
local lastCameraRotation = 0
RunService.Heartbeat:Connect(function(delta)
local _, yRotation, _ = Camera.CFrame:ToOrientation()
weaponSway:Impulse((yRotation - lastCameraRotation) * delta * 0.05)
Weld.C0 = Weld.Part0.CFrame:Inverse()
Weld.C1 = (Weld.C1 * CFrame.Angles(0, weaponSway.Position, 0)):Inverse()
end)
Yeahh not sure it’s working as intended lol i just became superman for a sec.
Same script as what you put.
1 Like