So, I put together a AFK handler, basically when you tab out it edits your player header where it shows you’re AFK.
However, whenever someone goes AFK, it changes everyone’s titles to whoever went AFK first.
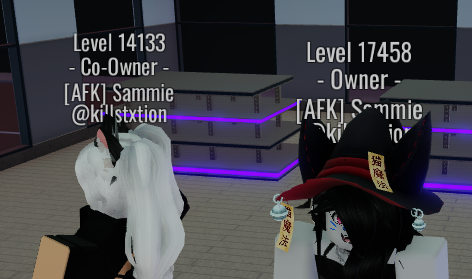
Here’s some code, SERVER SCRIPT:
-- AFK HANDLER --
AFKEvent.OnServerEvent:Connect(function(player,afk)
if afk then
header.Label3.Text = "[AFK] "..player.DisplayName
if player.DisplayName == player.Name then -- HIDES LABEL4 IF DOESNT HAVE A DISPLAY NAME
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then -- SHOWS LABEL4 IF HAS A DISPLAY NAME
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
else
header.Label3.Text = player.DisplayName
if player.DisplayName == player.Name then
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
end
end)
-- END OF AFK HANDLER
LOCAL:
--[ LOCALS ]--
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local AFKEvent = ReplicatedStorage.Remotes.AFK
local UserInputService = game:GetService("UserInputService")
--[ FUNCTIONS ]--
game:GetService("UserInputService").WindowFocusReleased:Connect(function() -- tab out
AFKEvent:FireServer(true)
end)
game:GetService("UserInputService").WindowFocused:Connect(function() -- tab in
AFKEvent:FireServer(false)
end)
Some background for context: The Server script block is within a module that handles the creation of the titles and everything related to those titles.
If you need more information just let me know.
The problem most likely lies in the header
variable, what is that defined as?
I’ll just paste the full module script that handles the header. this was just a function within the main function
function HeaderCreator.CreateTitle(player, developer)
local header = ReplicatedStorage.OverheadGui:Clone()
local groupRole = player:GetRoleInGroup(Configurations.GROUP_ID)
local level = player.player_stats.Level
local locked = false
header.Label.Text = "Level ".. level.Value
header.Label2.Text = "- ".. groupRole .. " -"
header.Label3.Text = player.DisplayName
if player.DisplayName == player.Name then
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then
header.Label4.Text = "@"..player.Name
--header.Label4.Size = UDim2.new(1,0,1.5,0)
end
header.Size = UDim2.new(5,0,2,0)
if (player.Character:WaitForChild("Head"):FindFirstChild("OverheadGui")) then
player.Character.Head.OverheadGui:Destroy()
end
-- AFK HANDLER --
AFKEvent.OnServerEvent:Connect(function(player,afk)
if afk then
header.Label3.Text = "[AFK] "..player.DisplayName
if player.DisplayName == player.Name then -- HIDES LABEL4 IF DOESNT HAVE A DISPLAY NAME
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then -- SHOWS LABEL4 IF HAS A DISPLAY NAME
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
else
header.Label3.Text = player.DisplayName
if player.DisplayName == player.Name then
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
end
end)
-- END OF AFK HANDLER
header.Parent = player.Character:WaitForChild("Head")
if (connections[player.UserId]) then connections[player.UserId]:Disconnect() end
connections[player.UserId] = level.Changed:Connect(function(value)
header.Label.Text = "Level ".. value
header.Label2.Text = "- ".. groupRole .." -"
end)
end
Here’s what my guess is:
You seem to have 2 parameters that’s defined as the player
that fired the event, could you maybe change the CreateTitle
function to this?
function HeaderCreator.CreateTitle(Player, developer)
Lua is case-sensitive so that might be the issue, I could be wrong though
Hmm, that didn’t do anything I don’t think.
Should I try running the handler into a different script maybe? I’m still not sure why it’s changing it for everyone.
Try implementing your OnServerEvent
outside the CreateTitle
function?
function HeaderCreator.CreateTitle(player, developer)
local header = ReplicatedStorage.OverheadGui:Clone()
local groupRole = player:GetRoleInGroup(Configurations.GROUP_ID)
local level = player.player_stats.Level
local locked = false
header.Label.Text = "Level ".. level.Value
header.Label2.Text = "- ".. groupRole .. " -"
header.Label3.Text = player.DisplayName
if player.DisplayName == player.Name then
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then
header.Label4.Text = "@"..player.Name
--header.Label4.Size = UDim2.new(1,0,1.5,0)
end
header.Size = UDim2.new(5,0,2,0)
if (player.Character:WaitForChild("Head"):FindFirstChild("OverheadGui")) then
player.Character.Head.OverheadGui:Destroy()
end
header.Parent = player.Character:WaitForChild("Head")
if (connections[player.UserId]) then connections[player.UserId]:Disconnect() end
connections[player.UserId] = level.Changed:Connect(function(value)
header.Label.Text = "Level ".. value
header.Label2.Text = "- ".. groupRole .." -"
end)
end
-- AFK HANDLER --
AFKEvent.OnServerEvent:Connect(function(player,afk)
if afk then
header.Label3.Text = "[AFK] "..player.DisplayName
if player.DisplayName == player.Name then -- HIDES LABEL4 IF DOESNT HAVE A DISPLAY NAME
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then -- SHOWS LABEL4 IF HAS A DISPLAY NAME
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
else
header.Label3.Text = player.DisplayName
if player.DisplayName == player.Name then
header.Label4.Text = " "
elseif player.DisplayName ~= player.Name then
header.Label4.Text = "@"..player.Name
-- header.Label4.Size = UDim2.new(5,0,1.5,0)
end
end
end)
-- END OF AFK HANDLER
If I move the function out of the main function, it breaks and doesn’t actually do the thing.
I fixed it, I had to change the path of the header within the AFK handler so that it looks at the header that was created, instead of actually creating a new one for everyone.