i want to achieve a custom 3d platformer character controller replacing the humanoid but i dont really know where to start
the issue is i dont even know how to make a character controller script that replaces the humanoid
ive tried using linear velocity for the movement (to limit the amount of force) for the walkspeed
the code below is what i use for character controlling
RS.RenderStepped:Connect(function()
local rayToGround = Ray.new(char.Position, Vector3.new(0,-2,0))
local hit, position = game.Workspace:FindPartOnRayWithIgnoreList(rayToGround, {vis})
local distance = (char.Position - position).Magnitude
if distance < 1 then
pose = "Idle"
char.LinearVelocity.Enabled = true
char.VectorForce.Force = upForce
end
-- char.AlignPosition.Position = char.Position + Vector3.new(0, 1, 0)
vis:SetPrimaryPartCFrame(char.CFrame)
if moveVector == true and walking == true then
if walkW == 1 then
force = Vector3.new(camera.CFrame.LookVector.X, 0, camera.CFrame.LookVector.Z)
elseif walkS == 1 then
force = Vector3.new(camera.CFrame.LookVector.X, 0, -camera.CFrame.LookVector.Z * linearVelocity.VectorVelocity.Z)
end
char.BodyGyro.CFrame = CFrame.new(Vector3.new(), force)
end
end)
UIS.InputBegan:Connect(function(input)
if input.KeyCode == Enum.KeyCode.W then
walking = true
walkW = 1
linearVelocity.VectorVelocity = Vector3.new(0, 0, -20)
pose = "Walking_Pose1"
elseif input.KeyCode == Enum.KeyCode.S then
walking = true
walkS = 1
linearVelocity.VectorVelocity = Vector3.new(0, 0, 20)
pose = "Walking_Pose1"
elseif input.KeyCode == Enum.KeyCode.A then
walking = true
walkA = 1
linearVelocity.VectorVelocity = Vector3.new(-20, 0, 0)
pose = "Walking_Pose1"
elseif input.KeyCode == Enum.KeyCode.D then
walking = true
walkD = 1
linearVelocity.VectorVelocity = Vector3.new(20, 0, 0)
pose = "Walking_Pose1"
elseif input.KeyCode == Enum.KeyCode.Space then
linearVelocity.VectorVelocity = Vector3.new(0, 0, 0)
char.AssemblyLinearVelocity = Vector3.new(0, 30, 0)
pose = "Jump"
char.LinearVelocity.Enabled = false
char.VectorForce.Force = Vector3.new(0, 0, 0)
end
end)
Sorry for the late reply. If you want to make a custom character controller, you’re going to have to delete the character and literally script all the movement. Things that you’ll need to learn are; Vectors, LookVectors, UserInputService, Raycasting, and Camera Manipulation. There isn’t a main source for learning how to make stuff like this so it can be quite difficult. I also recommend that you put things like ground checks in a separate function to prevent confusion.
so do i do rays instead of touch and touch ended?, btw how would i be able to make a part float in the air kinda like replicating the hip height of the humanoid
For things such as checking if the character is on the ground or checking if they are facing as wall should be RayCasted. More general stuff like collecting a coin can use regular Touch events.
For making the part float you’ll just have to set the Y axis of the LinearVelocity to 0;
LinearVelocity.VectorVelocity = Vector3.new(INSERT_NUMBER,0,INSERT_NUMBER)
I haven’t figured out how to make it work for slopes. I myself am currently creating something similar to this. I’ll let you know if I ever figure out the solution.
1 Like
ok, tsym, should i make raycast modules? so i dont have to constantly make a new ray and other stuff and just only raycast and return the hit part?
well, I personally just create a new RayCast every time because sometimes I want other properties like the RayCast normal and other stuff. If you want to make a module then go for it! It depends on what you want to add.
what if its going up a slope?, and also why set the Y axis to 0 instead of setting the other axis to 0 and the Y axis to a number to make it float up a specific height so it wont hit the ground
As stated in one of my previous replies, I haven’t necessarily figured out how to make it function with slopes. Also, The Y axis is set to 0 to make it float. Setting it to any number higher will make it just fly up infinitely.
I’ve experimented with making a movement system using a variety of objects and came to a conclusion. If you want to make character controllers, it’s a lot easier to use VectorForces instead of LinearVelocitys. Also, if you’re going to use VectorForces, I recommend going to the “CustomPhyscicalProperties” of your character part and setting it to these values:
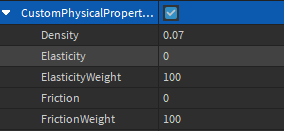