UPDATE VIDEO
Exmaple Place.rbxl (28.4 KB)
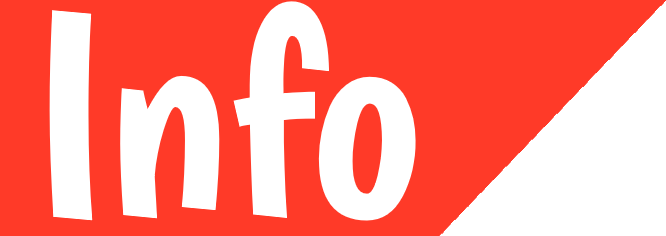
This is a service where you can create your own Audio Visualizer with ease. It allows you to customize your visualizer; whether is from making your Visualizer have textures to having a rainbow color bars. I hope this helps

Here is a video - Example Video
What is a Audio Visualizer?
An Audio Visualizer is model or a sort of object where it moves according to the audio frequency. Or in other words Parts moving according to the music.
How will the visualizer work?
We be using local script to control the movement of the visualizer. This will reduce lag in the movements. First we use the
Sound
Instance’s classPlaybackLoudness
to move the bars.
Is there any easier way to write this script?
Well In the future I will make a plugin for this project where it will have more intuitive way of customizing and creating an Audio Visualizer
- First Insert a LocalScript inside StarterPlayer.
- Download the Audio Visualizer Module or your use
require(5637113345)
to get the updated version
2.1 If you downloaded the Module then place in Replicated Storage - You can paste this Example Code in the LocalScript for test
Example Code
local Module = require(game.ReplicatedStorage.AudioVisualizerModule)
local RunService = game:GetService("RunService")
local Configs = {
SizeDirection = Enum.NormalId.Top,
PartType = Enum.PartType.Block,
StartingOrientation = Vector3.new(0,0,0),
Material = Enum.Material.Neon,
CustomPartName = "Part",
StartPosition = Vector3.new(0,0,0),
StartingSize = Vector3.new(3,3,0),
EndPoint = workspace.Visualizer,
Spacing = Vector3.new(4,0,0),
CloneNumber = 20,
LoudnessDivider = 15,
Music = workspace.Visualizer.Sound
}
Module.Create(Configs)
game.Workspace.Visualizer.Sound:Play()
RunService.RenderStepped:Connect(function()
for i,v in pairs(game.Workspace.Visualizer:GetChildren()) do
if v:IsA("Part") then
Module.Play(game.Workspace.Visualizer.Sound)
end
end
end)
Module.RainbowEffect(game.Workspace.Visualizer:GetChildren())
API
.Create()
This method would need Table value with your Configurations. This will create a Visualizer depending on your Configurations
Table Value needed
local Configs = { -- your Table Variable SizeDirection = Enum.NormalId.Top, -- MUST BE A ENUMITEM PartType = Enum.PartType.Block, -- MUST BE A ENUMITEM StartingOrientation = Vector3.new(0,0,0), -- MUST BE A INSTANCE VECTOR3 Material = Enum.Material.Neon, -- MUST BE A ENUMITEM CustomPartName = "Part", -- MUST BE A STRING StartPosition = Vector3.new(0,0,0), -- MUST BE A INSTANCE VECTOR3 StartingSize = Vector3.new(3,3,0), -- MUST BE A INSTANCE VECTOR3 EndPoint = workspace.Folder, -- MUST BE A INSTANCE VALUE AND IT MUST BE A FOLDER Spacing = Vector3.new(4,0,0), -- MUST BE A INSTANCE VECTOR3 CloneNumber = 20, -- MUST BE A INSTANCE INTEGER/NUMBER LoudnessDivider = 15, -- MUST BE A INSTANCE INTEGER/NUMBER Music = workspace.Visualizer.Sound -- MUST BE A INSTANCE VALUE }
.Edit()
This method would need Table value with your Configurations. It will create a new visualizer depending on your configurations, and will delete the old Visualizer
Table Value needed
local Configs = { -- your Table Variable SizeDirection = Enum.NormalId.Top, -- MUST BE A ENUMITEM PartType = Enum.PartType.Block, -- MUST BE A ENUMITEM StartingOrientation = Vector3.new(0,0,0), -- MUST BE A INSTANCE VECTOR3 Material = Enum.Material.Neon, -- MUST BE A ENUMITEM CustomPartName = "Part", -- MUST BE A STRING StartPosition = Vector3.new(0,0,0), -- MUST BE A INSTANCE VECTOR3 StartingSize = Vector3.new(3,3,0), -- MUST BE A INSTANCE VECTOR3 EndPoint = workspace.Folder, -- MUST BE A INSTANCE VALUE AND IT MUST BE A FOLDER Spacing = Vector3.new(4,0,0), -- MUST BE A INSTANCE VECTOR3 CloneNumber = 20, -- MUST BE A INSTANCE INTEGER/NUMBER LoudnessDivider = 15, -- MUST BE A INSTANCE INTEGER/NUMBER Music = workspace.Visualizer.Sound -- MUST BE A INSTANCE VALUE }
.Destroy()
This method would need the folder of your Audio Visualizer Folder. NOTE: It must be a Instance
local AudioVisualizerService = require(game.ReplicatedStorage.AudioVisualizerService) AudioVisualizerService:Destroy(workspace.Folder) -- Will Destroy your Visualizer
.AddColor()
This method would need a table value. This is help to find of the color should be Solid, Rainbow, have Texture, or All of them.
local ColorType = Configs["ColorType"] -- MUST BE A STRING AND EITHER "CUSTOM OR RAINBOW" local Color = Configs["Color"] or nil -- MUST BE A COLOR3 local Texture = Configs["Texture"] or nil -- MUST BE STRING OR NUMBER
.AddTexture()
This method would need the first Parameter to be an Instance or in this case the part. The second Parameter to be a Texture URL or ID. NOTE: Make sure this method is run under a for loop.
local AudioVisualizerService = require(game.ReplicatedStorage.AudioVisualizerService) for i,v in pairs(workspace.Folder) AudioVisualizerService:AddTexture(v,"YOUR ID") -- Will a Texture to your Visualizer on all sides end
.RainbowEffect()
This method would need the table value of all your parts. This method can be used in any other project outside of this Audio Visualizer Project.
local AudioVisualizerService = require(game.ReplicatedStorage.AudioVisualizerService) AudioVisualizerService:RainbowEffect(game.Workspace.Folder:GetChildren())
.Play()
This method would need first parameter to be the Sound Instance, the second to be the Parts Table, and the third is the Size Multiplier. This method can be use for any Audio Visualizer Project, this method is made so any Audio Visualizer is compatible with this method. NOTE: This method should be called every time the sound is played.
local AudioVisualizerService = require(game.ReplicatedStorage.AudioVisualizerService) AudioVisualizerService:Play(game.Workspace.Visualizer.Sound,workspace.Visualizer:GetChildren(),10)
1.0 - August 31, 2020
- Launch
- Bug Fixes
2.0 - September 2, 2020
- More Shapes Support
- Better Tweening and smoother animation
- More customization
- You can use Custom visualizer and connect to the
.Play()
method
FEEDBACK
Please reply to this post, it would help me a lot. You can comment on my work, or I need to fix any bugs, or even just want help. I will be updating the module.
Thanks for reading and hope this helped !!!