The provided code is a bit large - I recommend making a more minimal reproduction script, such as creating a new baseplate and adding the minimal amount of code needed to reproduce that bug.
local Players = game:GetService("Players")
local ClientCast = require(game:GetService("ServerScriptService").ClientCast)
local RemoteEvent = game:GetService("ReplicatedStorage").RemoteEvent
local Table = {} -- Player = Caster
local function StartCasting(Player)
Table[Player].Caster:Start()
task.wait(10)
Table[Player].Caster:Stop()
end
Players.PlayerAdded:Connect(function(Player)
Player.CharacterAdded:Connect(function(Character)
local Humanoid = Character:WaitForChild("Humanoid", 1)
local ClientCaster = ClientCast.new(workspace.ExamplePart1, RaycastParams.new())
ClientCaster:SetOwner(Player)
ClientCaster:StartDebug()
ClientCaster.HumanoidCollided:Connect(function(RaycastResult, HitHumanoid)
print("Hit model:" .. HitHumanoid.Parent.Name)
end)
Table[Player] = {Caster = ClientCaster}
Humanoid.Died:Connect(function()
Table[Player].Caster:Destroy()
task.wait()
Table[Player] = nil
end)
end)
end)
RemoteEvent.OnServerEvent:Connect(StartCasting)
This is the smallest I could get while keeping the structure relatively the same (hopefully that’s enough). This still causes the same error I posted above whenever the player dies and gets a new table entry and caster. This code is also no longer in a Module Script, so it’s not caused by that either. I also noticed now that the error only appears when the HumanoidCollided event is fired, not when casting starts or stops.
At what intervals is the remote fired? Could you replace it with a manual loop?
The remote was fired manually by me via UserInputService on a local script, but here’s the same script with a loop instead:
local Players = game:GetService("Players")
local ClientCast = require(game:GetService("ServerScriptService").ClientCast)
local RemoteEvent = game:GetService("ReplicatedStorage").RemoteEvent
local Table = {} -- Player = Caster
local function StartCasting(Player)
while Table[Player] ~= nil do
print("Started casting")
Table[Player].Caster:Start()
task.wait(5)
if Table[Player] ~= nil then
print("Stopped casting")
Table[Player].Caster:Stop()
task.wait(5)
end
end
end
Players.PlayerAdded:Connect(function(Player)
Player.CharacterAdded:Connect(function(Character)
local Humanoid = Character:WaitForChild("Humanoid", 1)
local ClientCaster = ClientCast.new(workspace.ExamplePart1, RaycastParams.new())
ClientCaster:SetOwner(Player)
ClientCaster:StartDebug()
ClientCaster.HumanoidCollided:Connect(function(RaycastResult, HitHumanoid)
print("Hit model:" .. HitHumanoid.Parent.Name)
end)
Table[Player] = {Caster = ClientCaster}
task.spawn(function()
StartCasting(Player)
end)
Humanoid.Died:Connect(function()
Table[Player].Caster:Destroy()
task.wait()
Table[Player] = nil
end)
end)
end)
For some reason this doesn’t cause the same error. Unfortunately that’s not exactly helpful to me, as I can’t rewrite the script without knowing what even causes the error in the first place…
v1.13.0
- Remove
ClientCaster:GetPing
- Remove
ClientCaster:GetMaxPingExhaustion
- Remove
ClientCaster:SetMaxPingExhaustion
-
- Sidenote: You should use
Player:GetNetworkPing()
now instead.
- Sidenote: You should use
- Update raycast data serialization to take up less space
I read all the introductions and comments, but found that only attachment can be used instead of bone. It’s a pity. I look forward to adding bones
Hey! Adding support for bones is definitely possible; I’ll look into it in the nearby future.
God, I’m so glad that you added this so soon. I tried to use RaycastHitboxV4 before. As you said, it also has server latency problems. I will use the new version immediately and give you feedback. Thank you for updating so quickly
Hi, my friend, did you submit the wrong version? I don’t think the code supports bone. In the OnDamagePointAdded function, there is only Attachment
hi,friend,I changed your module to support bones, and then tested it in the game. I think ClientCast is better than the RaycastHitboxV4 (because the Animation Backtracks of your module will not have much server delay, and the performance is very good,This should be the best melee attack system I’ve ever used). I hope you can continue to optimize
Bone
is a sub-class of Attachment
, and in the update I changed from .ClassName == "Attachment"
to :IsA("Attachment")
. This change should allow for using Bones also - did you make sure to rename the Bone to DmgPoint
?
I downloaded version 1.14.0 (release. rbxm). You can’t directly use the method of modifying the bone name (roblox’s bone system does not allow the existence of bones with the same name). I think you misunderstood my meaning, or I didn’t find the relevant use method (because I didn’t see any code related to bones in the module). I hope to add bones like Attachment (similar to RaycastHitboxV4), but it doesn’t matter, I modified the following functions OnDamagePointAdded and UpdateAttachment in a clumsy way (the same as the bone tracking of RaycastHitboxV4). My modifications and character bones are shown in the following figure (but I didn’t add the code related to the debug, so I can’t see the track of the related bones. After my test, the results are good):
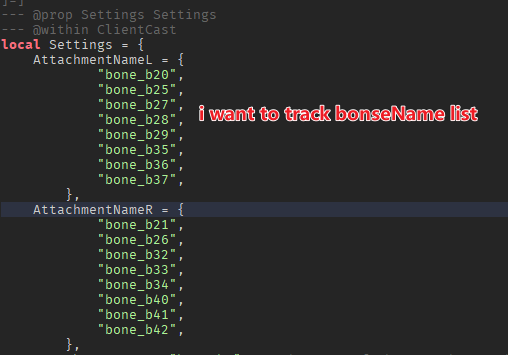
Ah, I see. I wasn’t aware you wanted to use bones without changing their name - I’ll consider making bones work with attributes too, and not just names.
Ok, friends, I’m looking forward to your update. I think in the near future, your module should be used by more developers who make action games
I have no clue wether is this a bug or not I’m attempting to use the example given everything runs fine but the humanoidcollided event is not firing whatsoever
Yeah me too, it just won’t fire for some reason.
For some reason both the collided and humanoid collided won’t fire.
Debug works fine, everything is good except for this.
Do you have any reproduction steps, ideally with a minimal amount of code? The following test place works for me:
TestPlace.rbxl (56.9 KB)