I looked a lot for a solution like this, but I didn’t find it. After much research and chats, I created a function that facilitates the creation of grids to be placed on the floor, for example, in creative games where the player wants to position an object inside a grid using a specific snap.
Here the grid decal I created in Photoshop:
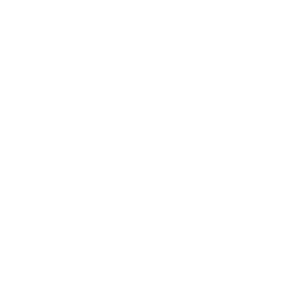
Here the script:
function CreateGrid(gridSizeX, gridSizeZ, gridPartDiameter, gridStartPosX, gridStartPosZ)
local gridFolder = Instance.new("Folder", workspace) -- to store all created grid blocks
gridFolder.Name = "GridFolder"
for x = gridStartPosX, (gridSizeX + 1) * gridPartDiameter, gridPartDiameter do
for z = gridStartPosZ, -gridSizeZ * gridPartDiameter, -gridPartDiameter do
-- create a new gridPart instance
local gridPart = Instance.new("Part", gridFolder) -- create the a grid part inside the gridFolder
gridPart.Size = Vector3.new(gridPartDiameter, 1, gridPartDiameter) -- the grid part will be a square
gridPart.Transparency = 1 -- hide the part
gridPart.Anchored = true
gridPart.CanCollide = false
gridPart.Name = string.format("%0.3i", x).."_"..string.format("%0.3i", z).."_grid" -- (not necessary) name the gridPart
gridPart.Position = Vector3.new(x, -0.5, z) -- position the grid under the ground (BasePlate)
-- create a Decal for gridPart
local gridDecal = Instance.new("Decal", gridPart) -- the decal image to be on the top of the gridPart
gridDecal.Texture = "rbxassetid://5095516829" -- my grid image (can be anything)
gridDecal.Face = "Top" -- put the decal image on top of the gridPart
end
end
end
-------------------------- Here you put your parameters ----------------
local SIZE_X = 3 -- width of the grid
local SIZE_Z = 6 -- depth/lenght of the grid
local PART_DIAMETER = 3 -- size of each grid block
local START_POS_X = 5 -- start position of the grid in the 3D scene
local START_POS_Z = -3
CreateGrid(SIZE_X, SIZE_Z, PART_DIAMETER, START_POS_X, START_POS_Z) -- create the grid
With the parameters of the example above, a grid of 6x3 blocks will be created, with initial position 5, -3, with each block having a width and length of 3 studs: