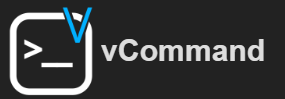
PLEASE RECOMMEND NEW FEATURES!
More Info: [HELP NEEDED] vCommand Admin Panel - #20 by oddcraft18
Welcome to vCommand Admin Panel! vCommand is a modular admin panel designed to help you moderate, modify, and control your experiences in real time in-game.
The home page of vCommand
Use vCommand to modify experiences at runtime and moderate against bad actors! You can also use gear to make advanced modifications to your experience.⚙️ Setup
- Get the model here or here.
- Place it in StarterGui
- Edit permissions in the PermedUsers ModuleScript.
- Optionally add extra Command Packs
Once you do that, press [ to open the menu. The fly keybind is E. You can run commands either by using the GUI or by chat commands.
Chat Command Formatting
Precise:
/commandPack command arguments
Extra Precise:
/commandPack,command,arguments
Imprecise:
/command arguments
Super Imprecise:
/command
📦 Packs
To install a pack, add it to the CommandPacks folder.To use packs, you’ll need a ModuleScript. All packs need a configuration named PackMeta, with a string attribute named Name. It should also have a Number attribute named Icon, a Boolean attribute named ColoredIcon, and a Number attribute named Power. Packs should go in the CommandPacks folder.
List of required pack components:
- ModuleScript
- PackMeta
- GUI
To make a pack, start by making it’s UI. In the SafeZone frame, there’s a frame called BlankTemplate. Build your UI in a duplicate of that. When your UI is complete, disable the Visible property and add it to the pack. The highest frame of the custom UI should be named Light. Then, adapt your UI for dark mode, and name it Dark. (You no longer need to adapt it for dark mode, dark mode is removed)
Then, you can begin coding the commands. When making a command, add it to the ModuleScript as a function, making sure the function is in the module table. The arguments have to be this: plr:Player, args
. You can name the arguments anything, but the first argument is a player and the second argument is a table that can be used to hold multiple arguments.
Once you’re satisfied with the commands, you should create the client-side interface. To run a command, fire the command remote. Here’s an example command in an example pack: Command:FireServer("ExampleCommandPack", "warn", {"Hello, World"})
. Argument 1 is the command pack, argument 2 is the command, and argument 3 is the argument table. Also, one last thing. vCommand comes with a built in name interpreter, so use it!
You can use anything from the default packs as reference or as a part of your own pack. I have also included template packs, feel free to use those!
🛡️Ranks
Ranks only need a few things. To create a rank, you need these properties in the returned table:- Name
- PowerLevel
- OthersCommands
The latter two control permissions. Disable OthersCommands to create a VIP-like rank. It can only run commands on itself. PowerLevel determines what packs a rank can use. To use a pack, a rank needs a power level at or above the level set in the pack.
Update Log
Packs Release
Added packs to vCommand and migrated all existing commands to packsModeration Pack
Added a moderation pack to vCommandIcon Update
Migrated all icons to Fluency Icon Pack and added sidebar icons to packs. They can be colored or monochrome.TopBarPlus Support
vCommand now supports TopBarPlus! If you're a mobile player, you can now use this admin panel! Added FreeForAll to permissions and slightly bugfixed default fly.Dark Mode
Added dark mode and fixed the vCommand Icon. TopBarPlus icon still isn't working.Bugfixes and Improvements
Fixed some bugs and added a built-in name parser.Commands Update
Added more player commands as well as fixed fly. Also removed dark mode.Mini Update!
You now stay vanished after dying.Chat Commands
Added chat commands to vCommand! Also added the Noclip command. Also I added the pull command sometime without mentioning it so... yeah... You can now pull players from any server into your own.Super Precise Chat Commands
You can now use commas to explicitly state each part of the command! You can now use commands with spaces in their name and use spaces in arguments!Lock and Shutdown Command!
You can finally lock and shut down servers using vCommand!New Gears
Added phone, bouncy ball, and apple pie gears.Ranks Update
Added modular ranks to vCommand. You can now control player ranks using vCommand.UI UPDATE!
Added window dragging, new open/close animation, and animations for switching packs!Packs Boost!
Added template packs and the Fly2 command!Bugfixes and Improvements
Fixed a lot of the errors so you don't get a wall of errors on unpublished places. Also, disables moderation pack on unpublished places. This update adds a quality monitor to make sure the CanvasGroup doesn't get blurry. Also makes dragging better.Rate vCommand
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
0 voters
- Yes
- No
0 voters
Please create a post with why you like/dislike vCommand.
Scripts
Main command hander:
local perms = require(script.Parent.PermedUsers)
local CommandPacks = script.Parent.CommandPacks
script.Parent.Command.OnServerEvent:Connect(function(plr:Player, cmd:string, arg1, arg2, arg3)
if not perms.PlayerIsAdmin(plr) then
plr:Kick("EXPLOITER")
return
end
local Pack = tostring(cmd)
if not CommandPacks:FindFirstChild(Pack) then return end
local UsedCommandPack = require(CommandPacks:FindFirstChild(Pack))
if not UsedCommandPack[arg1] then return end
if perms.GetPowerLevel(plr) < CommandPacks:FindFirstChild(Pack).PackMeta:GetAttribute("Power") then
plr:Kick("Bad Permissions")
return
end
UsedCommandPack[arg1](plr, arg2)
end)
game.Players.PlayerAdded:Connect(function(plr)
plr.Chatted:Connect(function(msg)
if not perms.PlayerIsAdmin(plr) then
return
end
local Chat = string.split(msg, " ")
if msg.find(msg, ",") then
Chat = string.split(msg, ",")
end
if string.sub(Chat[1], 1, 1) ~= "/" then return end
local Pack = string.sub(Chat[1], 2, string.len(Chat[1]))
local Command = Chat[2]
local Args = Chat
table.remove(Args, 1)
table.remove(Args, 1)
local RunningImprecise = false
if not CommandPacks:FindFirstChild(Pack) then
local Found = false
for i,v in CommandPacks:GetChildren() do
if string.lower(v.PackMeta:GetAttribute("Name")) == string.lower(Pack) then
Pack = v.Name
Found = true
end
end
if not Found then
RunningImprecise = true
end
end
local UsedCommandPack = nil
if not RunningImprecise then
UsedCommandPack = require(CommandPacks:FindFirstChild(Pack))
end
if (not RunningImprecise) and (not UsedCommandPack[Command]) then
local Found = false
for i,v in UsedCommandPack do
if type(v) == "function" then
if string.lower(tostring(i)) == string.lower(Command) then
Command = tostring(i)
Found = true
end
end
end
if not Found then return end
end
if RunningImprecise then
for a,b in CommandPacks:GetChildren() do
for i,v in require(b) do
if type(v) == "function" then
if string.lower(tostring(i)) == string.lower(Pack) then
Command = tostring(i)
UsedCommandPack = require(b)
Pack = b.Name
Args = string.split(msg, " ")
if msg.find(msg, ",") then
Args = string.split(msg, ",")
end
table.remove(Args, 1)
end
end
end
end
end
if CommandPacks:FindFirstChild(Pack) and perms.GetPowerLevel(plr) < CommandPacks:FindFirstChild(Pack).PackMeta:GetAttribute("Power") then
return
end
UsedCommandPack[Command](plr, Args)
end)
end)
Game pack:
local module = {}
function module.Unanchor(plr:Player, args)
workspace:breakJoints(workspace:GetDescendants())
for i,v in workspace:GetDescendants() do
if v:IsA("BasePart") then
v.Anchored = false
end
end
end
function module.Gravity(plr:Player, args)
workspace.Gravity = tonumber(args[1])
end
function module.Time(plr:Player, args)
game.Lighting.ClockTime = tonumber(args[1])
end
function module.TrueSight(plr:Player, args)
for i,v in workspace:GetDescendants() do
if v:IsA("BasePart") then
v.Transparency = 0
end
end
end
module["System Message"] = function (plr:Player, args)
local Chat = game:GetService("Chat")
for i,v in game.Players:GetChildren() do
script.SendSystem:FireClient(v, Chat:FilterStringForBroadcast(args[1], plr))
end
end
return module
Gear pack:
local module = {}
function module.GrantGear(plr:Player, args)
if script.Gears:FindFirstChild(tostring(args[1])) then
script.Gears[tostring(args[1])]:Clone().Parent = plr.Backpack
end
end
return module
Players pack:
local module = {}
local NameInterpreter = require(script:FindFirstAncestorOfClass("ScreenGui").NameInterpreter)
function module.Kill(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.Health = 0
end
end
function module.Speed(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.WalkSpeed = tonumber(args[2])
end
end
function module.Fling(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.PlatformStand = true
v.Character.HumanoidRootPart.Anchored = true
v.Character.HumanoidRootPart.AssemblyLinearVelocity = Vector3.new(200, 300, 200)
v.Character.HumanoidRootPart.Anchored = false
end
end
function module.Character(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid:ApplyDescriptionReset(game.Players:GetHumanoidDescriptionFromUserId(game.Players:GetUserIdFromNameAsync(tostring(args[2]))))
end
end
function module.Remove(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character:Destroy()
end
end
function module.Respawn(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
if v.Character then
local OldPos = v.Character.HumanoidRootPart.CFrame
v.CharacterAdded:Once(function(char)
task.wait()
char.HumanoidRootPart.CFrame = OldPos
end)
v:LoadCharacter()
else
v:LoadCharacter()
end
end
end
function module.Trip(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.PlatformStand = true
end
end
function module.Damage(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid:TakeDamage(args[2])
end
end
function module.JumpHeight(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.JumpHeight = tonumber(args[2])
v.Character.Humanoid.UseJumpPower = false
end
end
function module.JumpPower(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.Character.Humanoid.JumpPower = tonumber(args[2])
v.Character.Humanoid.UseJumpPower = true
end
end
function module.Fly(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.PlayerGui.AdminPanel.CommandPacks.vCommandDefaultPlayers.ToggleFly:FireClient(v)
end
end
function module.Bring(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
v.Character:PivotTo(plr.Character:GetPivot())
end
end
end
function module.Invisible(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
for i,v in v.Character:GetDescendants() do
if v:IsA("BasePart") then
if v.Transparency ~= 1 then
v.Transparency = 2
end
end
end
end
end
end
function module.Visible(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
for i,v in v.Character:GetDescendants() do
if v:IsA("BasePart") then
if v.Transparency == 2 then
v.Transparency = 0
end
end
end
end
end
end
function module.Vanish(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v:FindFirstChild("Vanished").Value = true
end
end
function module.God(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
local NewFF = Instance.new("ForceField")
NewFF.Visible = false
NewFF.Name = "vCommandGod"
NewFF.Parent = v.Character
end
end
end
function module.Ungod(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
if v.Character:FindFirstChild("vCommandGod") then
v.Character.vCommandGod:Destroy()
end
end
end
end
function module.Explode(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
if not plr.Character then return end
for i,v in People do
if v.Character then
local NewFF = Instance.new("Explosion")
NewFF.Position = v.Character:GetPivot().Position
NewFF.Parent = workspace
end
end
end
function module.Noclip(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.PlayerGui.AdminPanel.CommandPacks.vCommandDefaultPlayers.ToggleNoclip:FireClient(v)
end
end
function module.Fly2(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v.PlayerGui.AdminPanel.CommandPacks.vCommandDefaultPlayers.ToggleFly2:FireClient(v)
end
end
return module
Moderation pack:
local module = {}
if game.GameId == 0 then
return module
end
local DataStoreService = game:GetService("DataStoreService")
local BanStorage = DataStoreService:GetDataStore("vCommandBans")
local NameInterpreter = require(script:FindFirstAncestorOfClass("ScreenGui").NameInterpreter)
local PermedUsers = require(script.Parent.Parent.PermedUsers)
function module.Ban(plr, args)
local People = NameInterpreter.InterpretName(args[1], plr)
local Chat = game:GetService("Chat")
for i,v in People do
local UserId = v.UserId
local success, fail = pcall(function()
BanStorage:SetAsync(UserId, Chat:FilterStringForBroadcast(args[2], plr))
if game.Players:FindFirstChild(v.Name) then
v:Kick("You are banned from this game! Reason: "..args[2])
end
end)
if fail then
error("Failed to ban user "..UserId.."!")
end
end
if not People[1] then
local UserId = game.Players:GetUserIdFromNameAsync(args[1])
local success, fail = pcall(function()
BanStorage:SetAsync(UserId, Chat:FilterStringForBroadcast(args[2], plr))
end)
if fail then
error("Failed to ban user "..UserId.."!")
end
end
end
function module.Unban(plr, args)
local People = NameInterpreter.InterpretName(args[1], plr)
for i,v in People do
local UserId = v.UserId
local success, fail = pcall(function()
BanStorage:RemoveAsync(UserId)
end)
if fail then
error("Failed to unban user "..UserId.."!")
end
end
if not People[1] then
local UserId = game.Players:GetUserIdFromName(args[1])
local success, fail = pcall(function()
BanStorage:RemoveAsync(UserId)
end)
if fail then
error("Failed to unban user "..UserId.."!")
end
end
end
function module.Kick(plr, args)
local Chat = game:GetService("Chat")
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
v:Kick(Chat:FilterStringForBroadcast(args[2], plr))
end
end
function module.Mute(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
if game:GetService("TextChatService").TextChannels:FindFirstChild("RBXGeneral") then
if game:GetService("TextChatService").TextChannels.RBXGeneral:FindFirstChild(v.Name) then
game:GetService("TextChatService").TextChannels.RBXGeneral[v.Name].CanSend = false
end
end
end
end
function module.Unmute(plr:Player, args)
local People = NameInterpreter.InterpretName(args[1], plr)
if not People then return end
for i,v in People do
if game:GetService("TextChatService").TextChannels:FindFirstChild("RBXGeneral") then
if game:GetService("TextChatService").TextChannels.RBXGeneral:FindFirstChild(v.Name) then
game:GetService("TextChatService").TextChannels.RBXGeneral[v.Name].CanSend = true
end
end
end
end
function module.Pull(plr:Player, args)
local MessagingService = game:GetService("MessagingService")
MessagingService:PublishAsync("vCommandPull", {args[1], game.JobId, game.PlaceId})
end
module["Server Lock"] = function(plr:Player, args)
game.Players.PlayerAdded:Connect(function(plr)
if not PermedUsers.PlayerIsAdmin(plr) then
plr:Kick("This server is LOCKED! Please rejoin!")
end
end)
end
function module.Shutdown(plr:Player, args)
local Kick1 = Instance.new("ParticleEmitter")
Kick1.TimeScale = 0/0
Kick1.Parent = workspace
Kick1:Emit()
for i,v in game.Players:GetPlayers() do
v:Kick("Server has been shut down.")
end
end
return module
Keybind:
local uis = game:GetService("UserInputService")
local windowfuncs = require(script.Parent.WindowFunctions) -- open and close window
local perms = require(script.Parent.PermedUsers) -- player permissions
local ReplicatedStorage = game:GetService("ReplicatedStorage")
uis.InputBegan:Connect(function(input, gameProcessed)
if not perms.PlayerIsAdmin(game.Players.LocalPlayer) then -- only open if you're an admin
return
end
if gameProcessed then -- Make sure you're not chatting
return
end
if input.KeyCode == Enum.KeyCode.LeftBracket then -- use the [ key to open the menu
-- Toggle menu
if script.Parent.Enabled then
windowfuncs.CloseAdmin()
else
windowfuncs.OpenAdmin()
end
end
end)
if perms.PlayerIsAdmin(game.Players.LocalPlayer) then
local TopBarPlus
-- Auto Install TopBarPlus
if not ReplicatedStorage:WaitForChild("TopbarPlusReference", 1) then
require(script.Icon)
script.Icon.Parent = ReplicatedStorage
else
script.NoSelect.Parent = ReplicatedStorage:FindFirstChild("TopbarPlusReference").Value.Themes
end
TopBarPlus = require(ReplicatedStorage:FindFirstChild("TopbarPlusReference").Value)
local Themes = require(ReplicatedStorage:FindFirstChild("Icon").Themes)
local NewIcon = TopBarPlus.new()
:setTheme(Themes.new_ui)
:setRight()
:setProperty("deselectWhenOtherIconSelected", false)
:setImage(13569464782)
NewIcon:bindEvent("selected", function()
NewIcon:deselect()
if script.Parent.Enabled then
windowfuncs.CloseAdmin()
else
windowfuncs.OpenAdmin()
end
end)
end
print("This game uses vCommand Admin Panel. Learn more at: ")
warn("https://devforum.roblox.com/t/vcommand-admin-panel/2392324")
Name Interpreter:
local module = {}
function module.InterpretName(name, from)
--VIP RANK LOGIC
if require(script.Parent.PermedUsers).GetPowerLevel(from) ~= 9999999 then
if not require(script.Parent.PermedUsers).GetRankStats(from).OthersCommands then
return {from}
end
end
local Username = nil
local FromName
if tostring(name) == "Instance" then
Username = name.Name
else
Username = tostring(name)
end
if tostring(from) == "Instance" then
FromName = from.Name
else
FromName = tostring(from)
end
if string.lower(Username) == "others" then
local result = {}
for i,v in game.Players:GetChildren() do
if v.Name ~= FromName then
table.insert(result, v)
end
end
return result
end
if string.lower(Username) == "all" then
return game.Players:GetChildren()
end
if string.lower(Username) == "me" then
return {module.NameToPlayer(FromName)}
end
local firstletter = string.lower(string.sub(Username, 1, 1))
local results = {}
for i,v in game.Players:GetPlayers() do
if string.lower(string.sub(v.Name, 1, 1)) == firstletter then
table.insert(results, v)
end
end
if results and string.len(Username) == 1 then
return results
end
results = {}
for i,v in game.Players:GetPlayers() do
if string.find(string.lower(v.Name), string.lower(Username)) then
table.insert(results, v)
end
end
if results then
return results
end
local lastattempt = module.NameToPlayer(Username)
if lastattempt then
return {lastattempt}
else
return {}
end
end
function module.NameToPlayer(name:string)
if game.Players:FindFirstChild(name) then
return game.Players:FindFirstChild(name)
elseif tostring(name) == "Instance" then
return name
else
return nil
end
end
return module
PermedUsers:
local module = {}
local admins = { -- add Usernames or UserIDs you want to have admin, along with their rank
{"user", "Admin"},
{1, "Moderator"}
}
local FreeForAll = true -- set to true to allow all users to use the admin panel
local Ranks = script.Parent.Ranks:GetChildren()
function module.PlayerIsAdmin(Player:Player)
for i,v in admins do
if Player.Name == v[1] or Player.UserId == v[1] then
return true
end
end
return FreeForAll
end
function module.GetPowerLevel(Player:Player)
if not module.PlayerIsAdmin(Player) then
return 0
end
local Rank = nil
for i,v in admins do
if Player.Name == v[1] or Player.UserId == v[1] then
Rank = v[2]
break
end
end
for i,v in Ranks do
if require(v).Name == Rank then
return require(v).PowerLevel
end
end
if not FreeForAll then
return 0
else
return 9999999
end
end
function module.GetRank(Player:Player)
for i,v in admins do
if Player.Name == v[1] or Player.UserId == v[1] then
return v[2]
end
end
return nil
end
function module.GetRankStats(Player:Player)
if not module.PlayerIsAdmin(Player) then
return nil
end
local Rank = nil
for i,v in admins do
if Player.Name == v[1] or Player.UserId == v[1] then
Rank = v[2]
break
end
end
for i,v in Ranks do
if require(v).Name == Rank then
return require(v)
end
end
return nil
end
return module
Scripts in vCommand as of 10/19/2023, 5:44 PM.