Ready for my superior knowledge of physics? (I am currently in a physics class, so hopefully I can help)
This is a situation where we would use what is called Kinematics. There are a couple main kinematics equations. I will put them here for reference
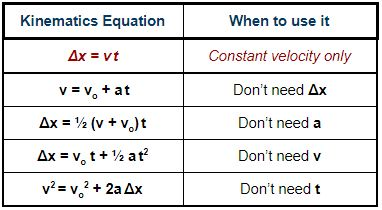
As shown by the picture, there are a few equations that would need to be used when you have specific information, but not enough. For your situation, we have the starting velocity, Vo, the acceleration, a. We also know the starting height. In order to have all of the necessary information, we need to take it in two parts. the vertical and horizontal.
In order to test it, we will assume that the initial velocity is Vector3.new(100, 250, 100)
, and the acceleration is going to just be Vector3.new(0, -workspace.gravity (196.2), 0)
. (All numbers are in studs per second)
For the vertical portion, we only need the Y component of the initial velocity. This gives us a starting velocity of 250 studs/sec and a velocity of -196.2, and a starting height of 0(above the baseplate) From there we start out with the equations.
(Visualization:)
we need to get the time that it will take for it to fall to the ground
WARNING: THIS ALL ASSUMES THAT THE LAND IS FLAT. IF IT IS NOT, MORE MATH IS INVOLVED
If that is the case, you can ping me, and I can help to flush out the kinks. (Basically you will need some raycasting to make sure that said final position is viable.)
From there we plug in the numbers to the “don’t need v(final velocity)” equation to get:
x = Vo * t + 1/2 * a * t^2
0 (starting height above baseplate) = 250 * t + 1/2 * (-196.2) * t^2
If you noticed, this is just a quadratic equation, so the quadratic formula needs to be used.
-98.1t^2 + 250t + 0 = 0
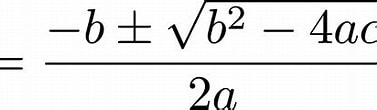
Sorry, it looks messy, idk how to format it
(250 (+-) sqrt(250^2 - 4(-98.1)(0)) / (2(-98.1)
We end up with 0, and 2.548… The 0 was already known–it started on the ground-- but now we know that it will take 2.548 seconds to hit the ground. With this, finding where it will hit is possible.
t = 2.548
Now we have the values needed: here they are:
Vo = V3(100,0,100)
V = not needed
x = ?
a = 0 (air resistance is negligable
t = 2.548
now, plug them into the “Constant Velocity Only” formula.
x = V3(100,0,100) * 2.548
x = V3(254.8, 0, 254.8)
It traveled 254.8 studs away in the x and z. That is going to be how far its position changed from before. For the actual position, you just add that to the original position.
Now for the juicy stuff… an actual script for this.
-- just add a new part into the workspace, and watch the magic happen
task.wait(3)
local function quadraticSolver(a, b, c)
local x1 = (-b + math.sqrt((b*b) -4 * a * c)) / (2 * a)
local x2 = (-b - math.sqrt((b*b) -4 * a * c)) / (2 * a)
-- usually going to be x2
return if x2 > x1 then x2 else x1
end
local function findLandingPosition(Vo: Vector3, startingPosition: Vector3)
local acc = -workspace.Gravity
local seconds = quadraticSolver((0.5 * acc), Vo.Y, startingPosition.Y)
local horizontalVel = Vector3.new(Vo.x, 0, Vo.Z)
local endingOffset = horizontalVel * seconds
return startingPosition + endingOffset + Vector3.new(0, -startingPosition.Y, 0)
end
local function createPartAtLandZone(pos: Vector3)
local part = Instance.new("Part")
part.Size = Vector3.new(1,1,1)
part.Shape = Enum.PartType.Ball
part.BrickColor = BrickColor.new("Really red")
part.Transparency = 0.5
part.CanCollide = false
part.Anchored = true
part.CFrame = CFrame.new(pos)
part.Parent = workspace
end
local part = workspace.Part
local initialVelocity = Vector3.new(10, 100, 0)
part.AssemblyLinearVelocity = initialVelocity
createPartAtLandZone(findLandingPosition(initialVelocity, part.Position))