Sure! Here’s my Explorer setup:
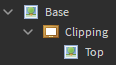
Here’s the Base ImageLabel, just set up as you usually would:

You can position and size it however you like. Nothing crazy about the image settings either:
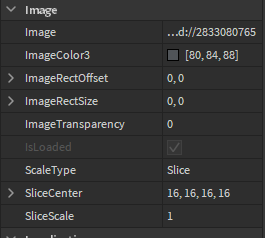
Inside that, I have a frame called Clipping:
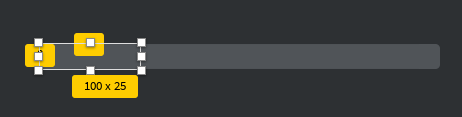
It’s just an invisible frame with ClipsDescendants enabled, and it’s sized to match the current progress. For example, if the current progress is 25%, the size of that frame will be {0.25, 0}, {1, 0}
, where the 0.25
represents the 25% as a decimal number between 0 and 1.
Inside of Clipping, I have the final ImageLabel, called Top:

You might notice by the selection box, Top is exactly the same size as Base. I suspect this is the issue with your code. To do this, we need to undo the sizing of Clipping.
This is simple enough! If the progress is 25%, we already know Clipping will have a scale of 0.25 horizontally, so to undo that scaling and get back to full width, we make Top have a scale of 4 horizontally. That’s because 0.25 * 4 = 1. Therefore, 1 / 0.25 = 4, so 1 / progress = the scale of Top!
Using that, we can figure out that, for a progress of 25%, the size of Top will be {1/0.25, 0}, {1, 0}
!
So how would that look in code? We can write a function to set the progress of the bar by applying all that math:
local Base = path.to.gui -- change this to refer to your Base ImageLabel
-- Set the progress of the bar
-- progress is a number between 0 and 1
local function SetProgress(progress)
local oneOverProgress
if progress == 0 then
-- since you can't do 1/0 (it's undefined), we'll just make oneOverProgress = 0
oneOverProgress = 0
else
oneOverProgress = 1/progress
end
Base.Clipping.Size = UDim2.new(progress, 0, 1, 0) -- set Clipping size to {progress, 0, 1, 0}
Base.Clipping.Top.Size = UDim2.new(oneOverProgress, 0, 1, 0) -- set Top size to {1/progress, 0, 1, 0}
end
Then, just call that function whenever you like and the progress bar should change! Of course, tweak it to fit whatever use case you may have.
As someone mentioned above, you can also tween the size of the progress bar if you want, just as long as you tween both the sizes of Clipping and Top at the same time, you should be fine!

Hope this helps 