I’ve actually made a system that forgoes all the channel stuff. It might help you out.
It’s a bit janky but It works in my testing so far:
Just copy the thing I made here:
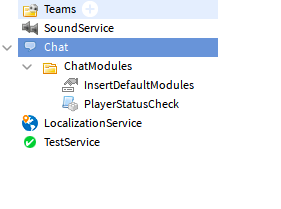
create a module script named playerstatuscheck as shown above, then paste the code i made down below in it. You will have to edit it to work with your role system though
Make sure insertdefaultmodules is a bool value with the value set to true
local module = {}
local function Run(ChatService)
local function ProcessMessage(speakerName, message, channelName)
local speaker = ChatService:GetSpeaker(speakerName)
local channel = ChatService:GetChannel(channelName)
if not speaker then return false end
if not channel then return false end
if game.Players[speakerName]:FindFirstChild("Role") then -- Check if they have a role
if game.Players[speakerName]:FindFirstChild("Role").Value == "dead" then -- is the role dead?
for i, plr in pairs(game.Players:GetPlayers()) do -- this loop will only send the message to other dead players
if plr:FindFirstChild("Role") then -- does the player were going to send the message to have a role?
if plr:FindFirstChild("Role").Value == "dead" then -- are they dead? if so send them the message
local speaker = ChatService:GetSpeaker(plr.Name)
if speaker then
speaker:SetExtraData("Tags",{{TagText = "Dead", TagColor = Color3.fromRGB(126, 126, 126)}})
speaker:SendMessage(message, channelName, speakerName, message.ExtraData)
end
end
else -- they have no role (there in the lobby), send the message
local speaker = ChatService:GetSpeaker(plr.Name)
if speaker then
speaker:SetExtraData("Tags",{{TagText = "Dead", TagColor = Color3.fromRGB(126, 126, 126)}})
speaker:SendMessage(message, channelName, speakerName, message.ExtraData)
end
end
end
else -- they must be alive if there role does not = dead
for i, plr in pairs(game.Players:GetPlayers()) do -- send the message to ALL players even dead ones
local speaker = ChatService:GetSpeaker(plr.Name)
if speaker then
speaker:SetExtraData("Tags",{{TagText = "Alive", TagColor = Color3.fromRGB(57, 255, 63)}})
speaker:SendMessage(message, channelName, speakerName, message.ExtraData)
end
end
end
return true
else -- they have no role, send the message to others without a role and dead players. not alive players
for i, plr in pairs(game.Players:GetPlayers()) do -- this loop will only send the message to other dead players
if plr:FindFirstChild("Role") then -- does the player were going to send the message to have a role?
if plr:FindFirstChild("Role").Value == "dead" then -- are they dead? if so send them the message
local speaker = ChatService:GetSpeaker(plr.Name)
if speaker then
speaker:SetExtraData("Tags",{{TagText = "Dead", TagColor = Color3.fromRGB(126, 126, 126)}})
speaker:SendMessage(message, channelName, speakerName, message.ExtraData)
end
end
else -- they have no role send it to palyers who also have no role or are dead
local speaker = ChatService:GetSpeaker(plr.Name)
if speaker then
speaker:SetExtraData("Tags",{{TagText = "InLobby", TagColor = Color3.fromRGB(255, 0, 12)}})
speaker:SendMessage(message, channelName, speakerName, message.ExtraData)
end
end
end
end
return true
end
ChatService:RegisterProcessCommandsFunction("swallow_shadow_ban_chat", ProcessMessage)
end
return Run