@LightBeamRays
I have found an issue with the new rollout and was wondering if it could be fixed because it has messed up parts of a game I’m working on.
The way voxel occupancy works with the new water has changed and doesn’t work the way that it used to. For example, with the code below, it used to create a skinny vertical pole of water.
local region = Region3.new(Vector3.new(0,0,0), Vector3.new(4,40,4))
region = region:ExpandToGrid(4)
local function create3dTable(size)
local ret = {}
for x = 1, size.X do
ret[x] = {}
for y = 1, size.Y do
ret[x][y] = {}
end
end
return ret
end
local material = create3dTable(Vector3.new(1,10,1))
local occupancy = create3dTable(Vector3.new(1,10,1))
for i = 1,10 do
material[1][i][1] = Enum.Material.Water
occupancy[1][i][1] = 0.2
end
game.Workspace.Terrain:WriteVoxels(region, 4, material, occupancy)
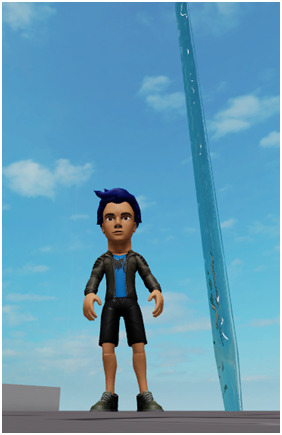
Now it doesn’t have any effect on the width of the vertical pole, but it does create a point at the top.
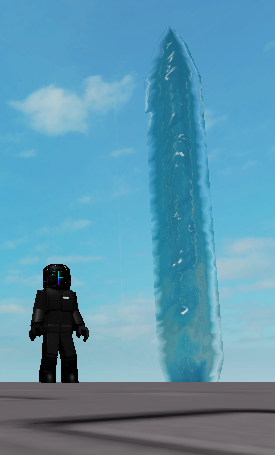
Also, in the past, if the code was changed so the pole extended sideways into the X or Z axis, instead of creating a skinny pole, it used to create a water blade like this. The example below is for a shorter version.
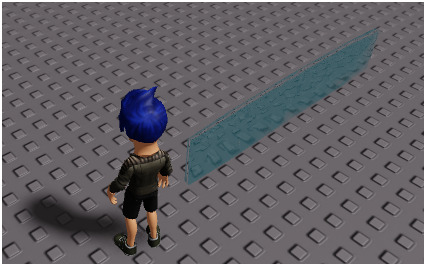
Now if I take the above code and extend it sideways in either the X or Z direction to create a water blade, it now creates a sideways pole instead.
local region = Region3.new(Vector3.new(0,0,0), Vector3.new(40,4,4))
region = region:ExpandToGrid(4)
local function create3dTable(size)
local ret = {}
for x = 1, size.X do
ret[x] = {}
for y = 1, size.Y do
ret[x][y] = {}
end
end
return ret
end
local material = create3dTable(Vector3.new(10,1,1))
local occupancy = create3dTable(Vector3.new(10,1,1))
for i = 1,10 do
material[i][1][1] = Enum.Material.Water
occupancy[i][1][1] = 0.2
end
game.Workspace.Terrain:WriteVoxels(region, 4, material, occupancy)
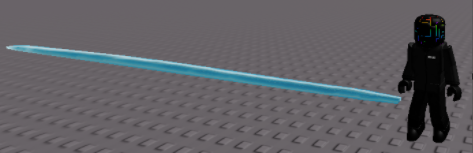
Although I’ve seen games with water blades which will be messed up if the water is converted to poles, I rather like the sideways poles now. But it would be good to be able to create both a blade or a pole.
What I really need is for the occupancy to be properly restored for the Y axis for vertical poles so I can create things like this again:
With the new water change, I have no flexibility at all over the width (occupancy) of the water in the Y direction. It has a single uniform thickness that can’t be altered except at the voxel level:
Can the occupancy of the Y axis please be fixed? And is it possible to have occupancy settings so that both blades and poles can be created?