v0.5.2 - Introducing Nature2D Plugins!
- Added new methods to Points
Point:SetMaxForce(maxForce: number)
- Added new methods to RigidBodies
RigidBody:SetMaxForce(maxForce: number)
- Added Plugins
- Quad
- Triangle
- MouseConstraint
So what are these “Plugins” all about? Don’t get confused, these plugins are not Roblox Studio plugins. These plugins are essentially utility modules that many people may find useful! I decided to create some of them for practices which are prominent, like creating custom RigidBody structures and being able to drag and move RigidBodies here and there using the mouse button.
There are 3 Plugins available as of now
- Quad - To create a 4 sided custom RigidBody structure given 4 points.
- Triangle - To create a triangular custom RigidBody structure given 3 points.
- MouseConstraint - A tool for dragging and moving RigidBodies using the mouse cursor.
In order to use plugins, you may require them in your script like this:
local ReplicatedStorage = game:GetService("ReplicatedStorage")
local Plugins = require(ReplicatedStorage.Nature2D.Plugins)
Example of the Triangle Plugin:
--[[
Plugins.Triangle(a: Vector2, b: Vector2, c: Vector2)
]]
local TriangleBody = Engine:Create("RigidBody", {
Mass = 5,
Structure = Plugins.Triangle(Vector2.new(10, 10), Vector2.new(10, 20), Vector2.new(0, 20)),
Collidable = true,
Anchored = false
})
Example of the Quad Plugin:
--[[
Plugins.Quad(a: Vector2, b: Vector2, c: Vector2, d: Vector2)
]]
local QuadBody = Engine:Create("RigidBody", {
Mass = 5,
Structure = Plugins.Quad(Vector2.new(0, 0), Vector2.new(20, 20), Vector2.new(0, 20), Vector2.new(20, 0)),
Collidable = true,
Anchored = false
})
Example of MouseConstraint:
--[[
Plugins.MouseConstraint(engine: Engine, range: number)
]]
Plugins.MouseConstraint(Engine, 10) -- RigidBodies that are closest to the mouse and under a radius of 10 pixels can be dragged around by holding and moving the mouse.
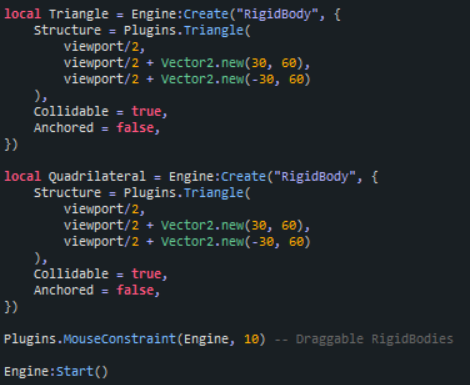
If you wish to see more plugins be added, do share some feedback with me. If you wish to have your plugins be added to Nature2D, open a pull request at the Github Repository!
Updated Roblox Asset & Github
Updated Documentation
Updated Wally Package - 0.5.1 → 0.5.2
Documentation up to the latest version will be updated soo !