Disclaimer: I will not explain the API of Rocrastinate, or its particulars in this post. Instead, I have a tutorial on GitHub explaining the particulars of Rocrastinate and how it works. I would also say that this library is for more advanced lua programmers. Please check out this link:
I just released a new UI library called Rocrastinate. Rocrastinate is a class-based approach to UI that offers similar declarative features to the roblox-endorsed libraries Roact/Rodux, but with much more versatility and optimization for the Roblox environment.
The core feature of Rocrastinate is that, unlike Roact, UI updates are âprocrastinatedâ until a UI component absolutely needs to be renderedâi.e. during a RenderStepped or Heartbeat binding. Rocrastinate guarantees that a UI element will be rendered at most once per frame. This, I find, is more suitable for Roblox development than Roact, as we donât need to reconcile a bunch of information every single time a portion of the state changes. We only need to do that just before we are displaying that information to the user; Roblox UI is bottlenecked by RenderStep, so I see no reason to handle these things asynchronously.
Finally, with my experience of using Roact, I believe it was too restrictive when working with UI templates. Roblox has a complex UI editor, and a great workflow for designing UI objectsâwhy should this be thrown away? With Rocrastinate, you have full control over how your UI is created and rendered, including the ability to :Clone() from templates.
Here is the composite of all of the UI I designed for a Rocrastinate project. These are static templates which are :Clone()'d at runtime.
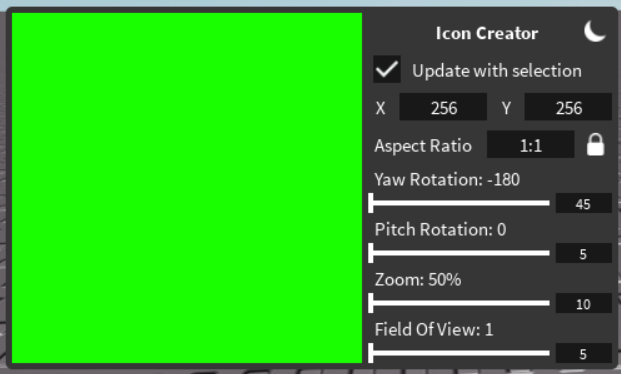
I made a plugin in order to test out the viability of the framework, and see if the workflow was one I liked. Overall, I can confidently say that this library offers a lot in terms of keeping code clean, re-usable, and scalable. I will be using Rocrastinate for my future projects.
Proof of Concept: Icon Creator plugin
Hereâs the tree structure of the Plugin I created with Rocrastinate:
Here are some key points of understanding this structure:
- You can see the modules âactionsâ and ârootReducerâ âthese are used for the Redux-like features of managing the pluginâs whole UI state
- The structure is similar to a React app, but with the added benefit that each Component renders Gui Templates, rather than creating each object by script and going through the costly process of reconciliation. With Rocrastinate, the minimal amount of rendering is applied when a component needs to update.
- You can see the pluginâs different portions are highly componentized.
The âAppâ module encapsulates the whole plugin while it is active.
The âPluginWindowâ encapsulates the main draggable frame in which the plugin can be used.
From there, you have âPropertiesPanelâ and âIconViewportâ (the two main components within the window), and these are further subdivided into re-used components like âNumericInputBoxâ, âSliderâ, and âBoolCheckboxâ
Video Link (ignore the cursor offset; thatâs just my screen recorder)
Plugin Link:
Icon Creator - Roblox
Place File:
https://raw.github.com/headjoe3/Rocrastinate/master/examples/icon_creator.rbxl
Check out the tutorial:
You can get the Rocrastinate library here:
This is just the initial release. For those who have used Roact in the past, I highly encourage you take a look at this library and see the results for yourself.