This is great! I loved
your old version of RotatedRegion3 and it’s been incredibly useful in many projects. Any time that I want to do collision detection I reach for this tool!
My favorite use case was a C&C Renegade-esque RTS/FPS project I worked on a while back for another person. Players could place buildings like in any other RTS, but they were big enough that players could walk into them! I needed to disallow placing buildings such that they overlap other buildings or the terrain (made of parts), so some kind of collision detection was needed.
I wanted to make it obvious to the player where they could or could not place any given building, so I decided to have a “ghost” version of a building showing where it will be placed, and to color the “ghost” red or green to show if it’s overlapping something:
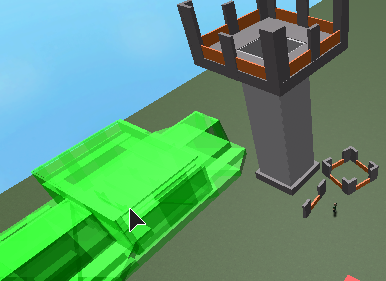
This means running the collision detection every frame, between several parts. To make this performant with many buildings and terrain consisting of 1000s of parts I used octrees (or quadtrees, don’t remember) to filter out things that were too far away to collide anyway. I also enclosed every building in a single part describing it’s bounding box (not axis aligned), to further filter out buildings that weren’t even close to touching, despite being physically close. Finally, the actual collision detection only needed to be run between 1 to 5 parts per building in the vicinity (5 for the most complicated buildings).
The tower in the pictures has a pretty big overhang, and I wanted to allow players to place small buildings beneath towers, as well as on top of or inside certain other buildings. Instead of just enclosing each building in a single volume that excludes other buildings, the “collision volume” can consist of any number of parts for fairly fine control.
Using these optimizations, we got the game to run with no drop in frame rate on my laptop (Intel HD Graphics 4000 and i5-3320M CPU @ 2.60 GHz). IIRC we didn’t do any actual benchmarks other than “yep, it runs at 60 fps”. For reference, my laptop can’t run Phantom Forces without occasionally dipping below 30 fps.
Anyway, I just wrote this to show an example of how this module can be super useful, and to show that it’s fine to do a few collision checks every frame. In a different game I’m just doing a super simple check to see if the player’s feet are touching the ground, so it’s also useful in simpler cases.