Having this weird quirk where the voxels just pause for a brief moment for some reason. This ruins the smooth feeling of the destruction and i have no clue how to fix it.
Look at the chunks and notice how they just dont move for a few frames before falling.
This is all im doing in the code:
1 Like
Seems like there is a bit of a delay when creating voxels on the server.
I tried your script on the client and it works fine:
But when it comes to doing it on the server, there is a delay like you said:
This is likely due to physics calculations on the server, specifically with applyImpulse being somewhat costly for performance, and you get that brief pause.
I recommend firing the table on the server back to all clients, destroying them on the server, then cloning them on the client, and the delay is gone:
Here’s the scripts for that as well:
--Local Script
local UIS = game:GetService("UserInputService")
local VoxBreaker = require(game.ReplicatedStorage.VoxBreaker)
local mouse = game.Players.LocalPlayer:GetMouse()
UIS.InputBegan:Connect(function(input,gpe)
if gpe then return end
if input.UserInputType == Enum.UserInputType.MouseButton1 then
local cframe = mouse.Hit
game.ReplicatedStorage.RemoteEvent:FireServer(cframe)
end
end)
game.ReplicatedStorage.RemoteEvent.OnClientEvent:Connect(function(voxels,cframe)
for _,voxel in voxels do
local clone = voxel:Clone()
clone.Parent = workspace
clone.Anchored = false
local velocity = CFrame.lookAt(clone.Position,cframe.Position).LookVector * (-50 * (clone.Mass))
clone:ApplyImpulse(velocity)
clone:ApplyAngularImpulse(velocity)
end
end)
--Server Script
local VoxBreaker = require(game.ReplicatedStorage.VoxBreaker)
local remote = game.ReplicatedStorage.RemoteEvent
remote.OnServerEvent:Connect(function(player,cframe)
local voxels = VoxBreaker:CreateHitbox(Vector3.new(10,10,10),cframe,Enum.PartType.Ball,4,30)
for _,voxel in voxels do
voxel.Parent = game.ReplicatedStorage --Parent voxels to replicated storage so that the client can see them
end
remote:FireAllClients(voxels,frame) --Fire the table back to the client before destroying them on the server
for _,voxel in voxels do
voxel:Destroy()
end
end)
3 Likes
I actually made this even more smooth by just doing it both on the client and server but making the server delete the voxels. This is slightly more faster and smoother because there is no cloning involved.
Honestly though props for this module. I was able to replicate the lag free destruction in Jujustu Shenanigans in only a few lines of code.
2 Likes
Yeah, it’s got nothing to do with the module. It’s just apply impulse. It’s an awful function that still has a long replication delay. This applies to all new movers too, like linear velocity. We are still, after many months, waiting for @DrCherenkov to fix this garbage.
1 Like
yeah I’m still relying on bodyVelocity for pretty much all use cases of moving parts. Pretty baffling to me how we still don’t really have any good alternatives.
1 Like
Yeah, he keeps claiming he’s “working with other developers so the change isn’t breaking”, but uh, dunno if he realizes this, currently, it’s broken for everyone. So, whoever these special cringelords that are apparently holding back the change because “waaah I can’t get my car from 2016 working using this system!!!” They should just update whatever ancient garbage they are holding onto instead of holding us all back.
2 Likes
I’ve been wanting to replicate Jujutsu Shenanigan’s destruction. Would you mind showing me how you’ve done this?
This is how I used the module to get my result. Take a look and see what you can take from it:
My result:
https://vimeo.com/950600011?share=copy
1 Like
when i try to do it on both the server and client the voxels just dont exist on the client
i dont know if its because im doing it on the client first, and then destroying them on the server without a wait or something though
if you can help id appreciate it
Try doing task.wait(.5)
before doing it on the server. I modified the timings in the module to prevent flickering so if it still doesn’t work for you I can send you my version.
thanks for the general server and client fix, i also changed like one time thing in my module so it didnt flicker, but i have this issue where the voxels just kinda keep getting smaller the more times the module is called
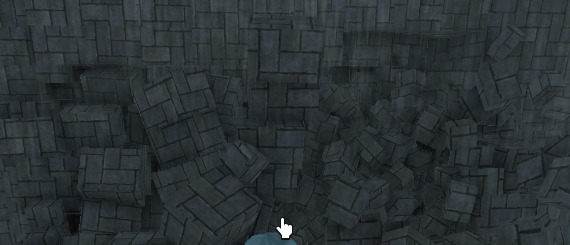
i dont really know how i would fix this because both im still kinda confused on how the module works and other stuff
if you have any solutions i think theyd be helpful
When looping through the voxels just do:
Voxel:SetAttribute("Destroyable", false)
Since the voxels are clones of the original part the destroyable attribute stays.
Or you can just make the minimum size bigger.
1 Like
well when i did that it somewhat fixed the problem with the voxels being smaller,
but now theres just excessive amounts of them like this:
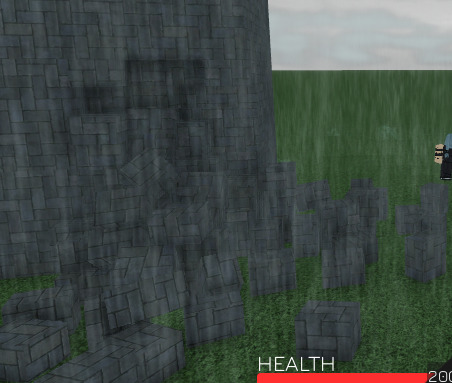
and sometimes the voxels are just smaller anyway

i can get a video if you want to see what im talking about
You should look back at the code I sent. I limited the amount of voxels that can come out per explosion by counting how many there are in the for loop and checking if its above the limit. If it is I just destroy the rest.
And for the sizes, I just set each voxel to a target size (for me 2 by 2 by 2 studs) then randomly add variation to them.
i didnt really want variation to my voxels, and my voxels keep getting smaller and smaller despite the minimum voxel size being set to 3
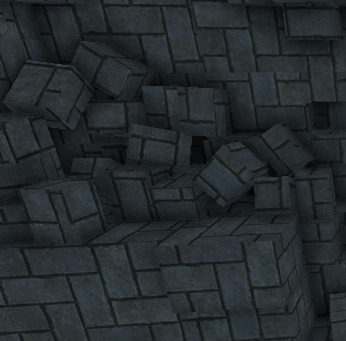
honestly if theres no solution to this can you just send your version
nvm i think i found a fix for this
is this another module you’re using?, and wouldn’t this lag if the voxels are created on the server?
No I am making a destruction module for my game using VoxBreaker. And I do the voxelization and the debris on the client then doing it on the server but deleting the voxels so the physics is only done on the client.
Then make it bigger and just set each voxel to be the exact size you want.