Alright so, I shall give an explanation
(And I’m on mobile so dear everything forgive me if the stuff I say isn’t formatted correctly)
A creator “value” is basically in simple terms, a Kill Check (Or detecting if there is a CreatorTag, then we can add a Kill stat to the player who defeated the target)
The way how the creator “value” is implemented, is that it’s basically a way of checking if a player’s Character
(Aka the Humanoid) has been damaged or not
Take a look at this GIF here:
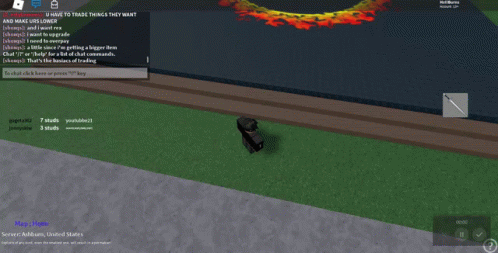
There are a couple things to note:
-
When the Sword touches a Character’s Model (Excluding the Player who welded it itself), it creates the “CreatorTag”, which is actually an ObjectValue
-
Upon a Character’s death (If a CreatorTag is in the target while he is dead in other words), that’s when the “CreatorTag” checks if the Character got tagged or not. If it is, then we can add a Kill to the Player who dealt the damage!
So how about we write just a simple code here, we’ll start with the tool that will deal damage:
local Tool = script.Parent
local Handle = Tool.Handle
local Damage = 10
Handle.Touched:Connect(function(Hit)
local Player = game.Players:GetPlayerFromCharacter(Hit.Parent)
if Player then
end
end)
If you don’t know how Tool functions work, I highly advise that you look at this here 
Anyways, so what the first thing we’re doing here is defining our variables, then creating our Touched
event, so that it fires whenever the tool hits a certain object (Or in this case, the Model of a Character)
local Player = game.Players:GetPlayerFromCharacter(Hit.Parent)
The next thing we’re doing here, is we’re actually getting the Player’s Instance from the Character’s Model itself (As Hit.Parent will refer to of course, the Hit’s Parent)
Adding onto our code here:
local Tool = script.Parent
local Handle = Tool.Handle
local Damage = 10
Handle.Touched:Connect(function(Hit)
local Player = game.Players:GetPlayerFromCharacter(Hit.Parent)
if Player then
Hit.Parent.Humanoid:TakeDamage(Damage)
local Tagged = Instance.new("ObjectValue")
Tagged.Name = "creator"
Tagged.Value = Player
Tagged.Parent = Hit.Parent.Humanoid
game.Debris:AddItem(Tagged, 2)
end
end)
Now we added some more things, but it shouldn’t be too hard to explain here:
Hit.Parent.Humanoid:TakeDamage(Damage)
This is referring to the Target’s Humanoid that will recieve the damage (OUCH)
local Tagged = Instance.new("ObjectValue")
Tagged.Name = "creator"
Tagged.Value = Player
Tagged.Parent = Hit.Parent.Humanoid
I’ll try to break this the best I can here:
-
Tagged.Name
is equal to the creator, it’s optional to change it but it’s good practice to learn
-
Tagged.Value
will actually refer to the Player, or in this case we’re getting the Player’s leaderstats upon triggered
-
Tagged.Parent
will head to the Target’s Humanoid, so that the Server Script (Will explain soon) will detect for any CreatorTags
So, now we have our weapon ready! But what’s next? Well, the last step is creating the Kill Check from the Server Side! This is to check for any potential CreatorTags lying around the Humanoid 
We’ll start with making the script inside ServerScriptService
, and we’ll mainly need to use the CharacterAdded event to fire every time a Character joins
game.Players.PlayerAdded:Connect(function(Player)
Player.CharacterAdded:Connect(function(Character)
Character.Humanoid.Died:Connect(function()
if Character.Humanoid:FindFirstChild("creator") then
local Player = Character.Humanoid.creator.Value
local Leaderstats = Player.leaderstats
Leaderstats.Kills.Value += 1
end
end)
end)
end)
Last stretch! Breaking down time (The majority of these will be events):
-
PlayerAdded
will fire whenever a Player joins the game, and CharacterAdded
will fire whenever a Character gets created into the Workspace
-
Character.Humanoid.Died
fires when the uhh, yes Character’s Humanoid reaches 0
(Inside that event, we’re also checking if we have a CreatorTag inside the Character’s Humanoid)
-
local Player
is actually referring to the Player Object we referenced back in our other Tool script, which was Tagged.Value = Player
-
local Leaderstats
refers to the Player’s local leaderstats held in a Folder
-
Leaderstats.Kills.Value += 1
is just simply, increasing the Player’s Kills by 1
Aaaaaand if you managed to reach the end, then congrats! You now have a basic understanding on what CreatorTags are hopefully
If you do have any more questions though, feel free to ask!
(AND SOMEONE PLEASE CORRECT ME IF I FORGOT SOMETHING CAUSE THIS TOOK WAY TOO LONG TO WRITE)