v0.5 - Custom RigidBody Support!
Major Release.
- Fixed
Engine:CreateCanvas()
- Canvas’ can now be re-initialized.
- Fixed
Constraint:Render()
- Prevent support constraints from rendering
- Added support for custom RigidBodies
- Added new Valid Property for RigidBodies -
Structure: table
- Updated Collision Detection and Response to work with custom RigidBodies
- Updated
Engine:Create()
- Restrict certain methods from being used for custom RigidBodies
You can now create more than just rectangles and squares. You can now define your own point-constraint structures like triangles, irregular quadrilaterals and n-sided polygons and then turn them into RigidBodies! Here’s how Custom RigidBodies work and how you can create your own!
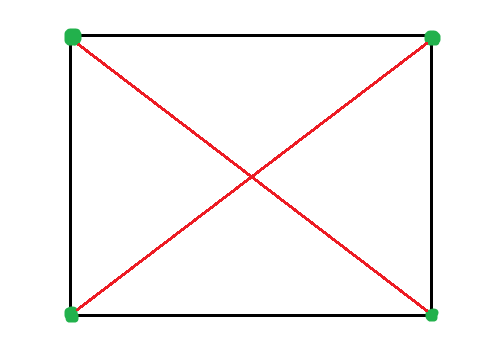
In the above image, the green dots are the arbitrary points we chose for our RigidBody, if we pass in a UI element, the 4 dots are automatically placed at its corners, also keeping in mind its rotation. There are 6 lines connecting the points all together. The lines in black are the edges of the RigidBody and the lines in red are “support constraints” which are meant to hold the RigidBody’s structure in place and it prevent it from collapsing into nothingness. It is worth noting that support constraints are not used in collision detection. All constraints are rods.
In order to create custom RigidBodies, you don’t need to specify a UI element/Object when using Engine:Create()
. You would pass in a property called “Structure”. This contains a table of constraint data. Here’s how a structure looks like for a rectangle:
-- { Point1: Vector2, Point2: Vector2, Support: boolean }
local struct = {
{ Vector2.new(0, 0), Vector2.new(0, 10) },
{ Vector2.new(0, 10), Vector2.new(10, 10) },
{ Vector2.new(10, 10), Vector2.new(10, 0) },
{ Vector2.new(10, 0), Vector2.new(0, 0) },
{ Vector2.new(0, 0), Vector2.new(10, 10), true },
{ Vector2.new(10, 0), Vector2.new(0, 10), true }
}
You just need to provide the positions of the points of the edges and specify if they are support constraints or not! Note that in order to create custom rigidbodies, you must specify a frame for the Engine’s Canvas. You can then use Engine:Create()
to turn this structure into a RigidBody. A simple example of a triangle:
local viewport = workspace.CurrentCamera.ViewportSize
local function MakeTriangle(a: Vector2, b: Vector2, c: Vector2)
return {
{ a, b, false },
{ a, c, false },
{ b, c, false }
}
end
local triangle = Engine:Create("RigidBody", {
Structure = MakeTriangle(viewport/2, viewport/2 + Vector2.new(-40, 80), viewport/2 + Vector2.new(40, 80)),
Collidable = true,
Anchored = false
})
Custom RigidBodies also support Quadtree collision detection! It is worth noting that not all methods will work for custom RigidBodies since the idea is fairly new. I will add the ability to use them in the future! If you try to use these methods, an error will pop up in the output window.
Updated Roblox Asset & Github
Updated Documentation
Updated Wally Package - 0.4.5 → 0.5.0
cc: @uhi_o