It works the same way on the server
What is the “physics load” in question? If you are talking about the roblox physics engine, you cannot make that parallel, roblox would have to do it themselves by adding a :SetThreadOwnership(), kind of like :SetNetworkOwnership()
Anyway, here is an example where I use it on the server, to deserialize a table that can get pretty big
local GameDatabase = Games[Task.TaskIndex]
if not GameDatabase then
local Data = DatastoreFunctions:GetGamesData(Task.TaskIndex) or tostring(GamesSerializer:GetLastestVersion())
Data = string.split(Data,"_")
local _version = tonumber(table.remove(Data,1))
local DataLenght = GamesSerializer:GetDataLenghtFromVersion(_version)
local GamesAmount = table.maxn(Data)/DataLenght
local Index = 0
for i = 0, Settings.ServerThreads -1 do
local Tasks = math.floor(GamesAmount/(Settings.ServerThreads-i))
GamesAmount -= Tasks
local a = Index + 1
local b = Index + Tasks*DataLenght
Index += Tasks*DataLenght
SerDeserModules.Games.Deser:ScheduleWork(table.concat(Data,"_",a,b),_version)
end
local FinalData = {}
Data = SerDeserModules.Games.Deser:Work()
for i, v in ipairs(Data) do
table.move(v,1,table.maxn(v),table.maxn(FinalData)+1,FinalData)
end
Data = nil -- TODO -- what the hell is happening here, why is this needed
local DataSize = table.maxn(FinalData)
Games[Task.TaskIndex] = {
Data = FinalData,
DataSize = DataSize,
Index = math.random(1,math.max(DataSize,1)),
}
GameDatabase = Games[Task.TaskIndex]
end
Here is the Deser module
local Settings = require(game.ReplicatedStorage.Settings)
local TasksPerThreads = Settings.SponsorsPerDatastore/Settings.ServerThreads
if TasksPerThreads ~= math.round(TasksPerThreads) then error("Invalid settings, cannot spread tasks evenly)") end
local Serializer = require(game.ServerScriptService.ServerTasks.TaskScript.Sponsors.SponsorsSerializer)
return function(String, _version, TaskIndex : number)
return Serializer:DecompressArray(String, _version)
end
the DecompressArray method is for decompressing multiple elements at once. I made my code to make it specifically Schedule work for every thread available (aka the number of actors, which is set in the settings for the module), instead of doing ScheduleWork for every element separately and having the ParallelScheduler merge them. It’s better performance wise to do it like this. It does complicate things a bit though
It is much simpler when not merging tasks together, though if you are getting into the territory of maybe 300-500 smallish tasks or more, you should merge them
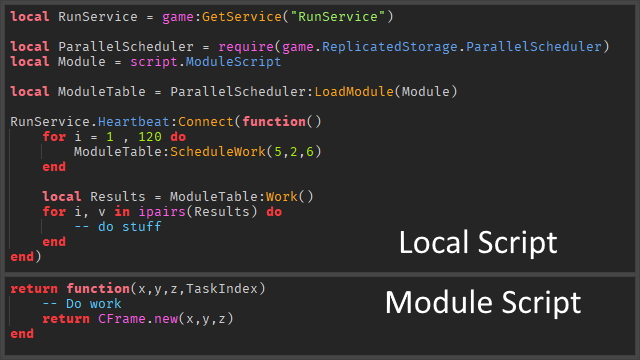