Quick note: this is meant for people who have little to no UI expierence so they can improve their games design.
This tutorial may no longer be needed because of the new AutoCanvasSize property - however this property has some issues as of now and I am not sure if it works in game or studio only.
Note: it is recommended that you don’t use “scale” for sizing your frames but instead “offset”.
Don’t you just hate it in games when you have an inventory GUI that is too small to fit all your items or is too big? Well, don’t be one of the developers who do that. Get all your scrolling frames to fit it’s content perfectly. No overscrolling, no underscrolling, just perfect.
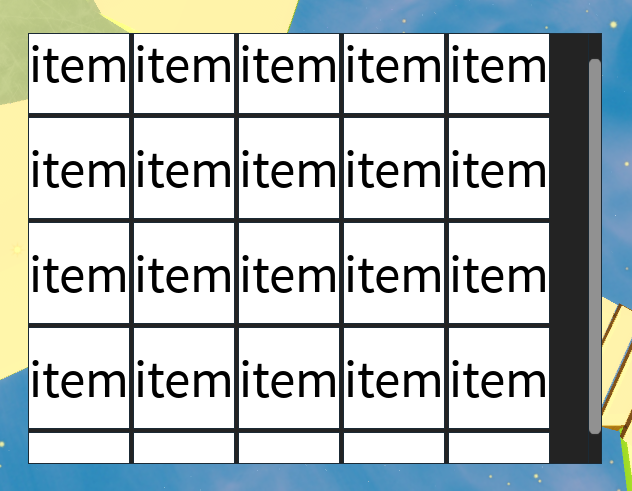
You can do this easily in a few basic steps, I will be going over how to do it with UI constraints, if you aren’t using UI constraints then I highly recommend using them.
Step 1: Getting the content size of your frame.
You can easily get the content size of your frame with the property under your List/GridLayout “AbsoluteContentSize”, this will return an X and a Y value.
Step 2: Setting your Canvas Size.
Now all you have to do is set your Canvas Size X and Y Offset to the Absolute Content Size values.
If you are using this for a constantly updating UI such as a player list or inventory then I would recommend making a function that you fire everytime those things update.
Example:
local function UpdateCanvasSize(Canvas, Constraint)
Canvas.CanvasSize = UDim2.new(0, Constraint.AbsoluteContentSize.X, 0, Constraint.AbsoluteContentSize.Y)
end
Just run this function whenever you want to update your canvas size, the “Canvas” argument is the scrolling frame and the “Constraint” Argument is the UI constraint.
If you are using this for something that isn’t constantly updating like a store GUI then you can either manually set it or set it via a script.
You are now ready to do me proud and make some proper scrolling frames, please do it. Improve your user expierence, please.